Discover How Machine Learning Can Amplify Your CodeIgniter Projects
As applications evolve, so do user expectations. The demand for smarter and more automated solutions is higher than ever. This is where machine learning (ML) enters the scene. Pairing machine learning with powerful PHP frameworks like CodeIgniter can make your application smarter, efficient, and way ahead of the curve.
CodeIgniter is a robust PHP framework known for its lightweight nature and speed. On the other hand, machine learning offers capabilities to predict, analyze, and automate tasks in unprecedented ways. Together, they can transform your applications from a traditional system to an intelligent one.
In this blog, we will delve into ways to integrate machine learning into a CodeIgniter application using practical examples.
1. Why integrate Machine Learning into CodeIgniter?
- Predictive Analysis: ML can predict user behavior and preferences, enabling your application to offer more personalized experiences.
- Automation: Automate tasks that were previously manual, thus saving time and reducing errors.
- Data Analysis: Dive deeper into your data to get insights that were not visible before.
Prerequisites:
– Basic understanding of CodeIgniter.
– Familiarity with machine learning concepts.
2. Predictive Analysis – Product Recommendations
Suppose you have an e-commerce site developed in CodeIgniter. You want to recommend products to users based on their previous purchases.
Steps:
- Collect Data: Store user purchase history in your database.
- Training a Model: Use a machine learning service or library to train a recommendation model. For this example, we’ll use a simple algorithm provided by a platform like TensorFlow or Scikit-learn.
- Integration: Once your model is trained, save the model and integrate it into your CodeIgniter application.
Integration Code:
```php class Recommendation_model extends CI_Model { public function get_recommendations($user_id) { // Load your trained model (e.g., model.pkl) $model = ... // Load model logic here // Fetch user's purchase history $purchase_history = $this->db->where('user_id', $user_id)->get('purchases')->result_array(); // Predict recommendations $recommendations = $model->predict($purchase_history); return $recommendations; } } ```
3. Automation – Customer Support Chatbot
Consider automating the initial interaction in your customer support with a chatbot.
Steps:
- Collect Data: Gather customer support interactions and categorize them.
- Train a Model: Use a machine learning platform, perhaps like Dialogflow or Rasa, to train a chatbot model.
- Integration: Implement the trained chatbot into your CodeIgniter application.
Integration Code:
```php class Chatbot_controller extends CI_Controller { public function get_response() { $user_message = $this->input->post('message'); // Communicate with your trained model (e.g., using an API endpoint) $response = ... // Get response from chatbot echo json_encode($response); } } ```
4. Data Analysis – User Behavior Insights
Let’s say you want to analyze how users navigate through your application and offer insights.
Steps:
- Collect Data: Log user interactions and page visits.
- Train a Model: Use a clustering algorithm to categorize user behaviors.
- Integration: Present insights on the admin dashboard.
Integration Code:
```php class Insights_model extends CI_Model { public function get_user_behaviors() { // Load your trained clustering model $model = ... // Load model logic here // Fetch user interaction logs $logs = $this->db->get('user_logs')->result_array(); // Get insights $clusters = $model->predict($logs); return $clusters; } } ```
Conclusion
Integrating machine learning into a CodeIgniter app can seem challenging, but the payoffs in terms of efficiency, automation, and predictive capabilities are immense. The examples we provided are basic and serve as starting points. In real-world scenarios, depending on the complexity of data and requirement, the approach may differ.
When implementing machine learning, always remember to continuously train your model with new data to keep the predictions relevant and accurate.
By embracing the combination of CodeIgniter and machine learning, you can propel your applications to new heights, offering enhanced user experiences and intelligent functionalities. Happy coding!
Table of Contents
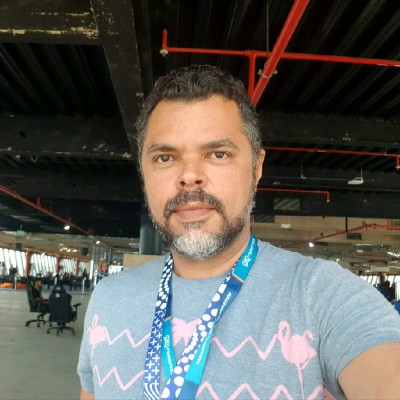
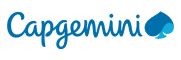