Crafting Web Masterpieces: Your Ultimate Guide to CodeIgniter and Microframeworks
In the world of web development, the choice of the right framework can significantly influence the efficiency, scalability, and manageability of the application. While there are several heavyweight frameworks, they might be overkill for many applications. This is where lightweight frameworks and microframeworks come into play. In this post, we’ll delve deep into CodeIgniter, a lightweight PHP framework, and discuss some other microframeworks that serve as great alternatives.
1. CodeIgniter
CodeIgniter is an open-source PHP framework known for its small footprint and outstanding performance. It’s a great tool for developers who want to build full-featured web applications without the overhead of larger frameworks.
2. Key Features of CodeIgniter
– Lightweight: The entire source code for CodeIgniter is only about 2MB, making it easy to master and deploy.
– MVC Architecture: It uses the Model-View-Controller (MVC) design pattern, which helps in separating logic from presentation.
– Loose Coupling: Components are loosely coupled, meaning you can replace or modify most parts of the framework without affecting others.
– Built-in Security Tools: CodeIgniter offers various tools for input and data handling, making it easier to write secure applications.
3. Building a Simple App with CodeIgniter
Here’s a quick demonstration of how to build a “Hello World” application using CodeIgniter:
- Download and install CodeIgniter from the official website.
- Navigate to `application/controllers`, and create a file named `Hello.php`.
- In `Hello.php`, add the following code:
```php <?php class Hello extends CI_Controller { public function index() { $this->load->view('hello_world'); } } ```
- Now, create a view for this controller. Navigate to `application/views` and create a file named `hello_world.php`.
- In `hello_world.php`, add:
```php <html> <head> <title>Hello World</title> </head> <body> <h1>Hello, World!</h1> </body> </html> ```
- Navigate to your project’s URL followed by `/index.php/hello` to see the “Hello, World!” message.
4. Microframeworks: What Are They?
Microframeworks are minimalistic web application frameworks that are often used for building small to medium-sized applications. They offer the essential tools and libraries needed for web development without the overhead of full-fledged frameworks. This means faster performance, easier learning curve, and greater flexibility.
5. Examples of Microframeworks
5.1. Lumen
Lumen is a PHP microframework developed by Laravel. It’s tailored for building microservices and lightning-fast APIs.
Example – Building a Simple API with Lumen:
```php // Installation composer require laravel/lumen // Create a new route in 'routes/web.php' $router->get('/greet', function () { return response()->json(['message' => 'Hello, World!']); }); ```
Access the `/greet` endpoint, and you’ll receive a JSON response.
5.2. Flask
Flask is a microframework for Python. It’s flexible and ideal for building small applications and APIs.
Example – Building a Simple App with Flask
```python from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run() ```
Run the script, and the application will start serving on the default port.
5.3. Sinatra
Sinatra is a DSL for quickly creating web applications in Ruby with minimal effort.
Example – Building a Simple App with Sinatra
```ruby require 'sinatra' get '/' do 'Hello, World!' end ```
Run the script with the Ruby interpreter, and your application will be up and running.
Conclusion
Whether it’s CodeIgniter or any of the aforementioned microframeworks, the goal remains the same: to provide developers with a platform to build applications without unnecessary overhead. By using these lightweight alternatives, developers can focus on building what’s essential, ensuring faster performance and quicker time to market.
Table of Contents
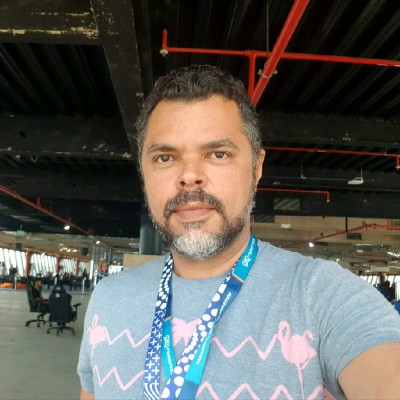
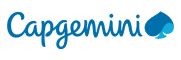