CodeIgniter and Mobile App Development: Building Native Apps
CodeIgniter, a popular PHP framework, is renowned for its simplicity and performance. Traditionally used for web application development, CodeIgniter can also play a role in mobile app development. This blog explores how CodeIgniter can be integrated with mobile app development frameworks to build native applications, leveraging its robust backend capabilities.
Why Use CodeIgniter for Mobile App Development?
Backend Efficiency
CodeIgniter excels in providing a lightweight and efficient backend. Its MVC architecture allows for clean and organized code, which is crucial for maintaining and scaling mobile applications. By using CodeIgniter for the backend, developers can ensure that the server-side logic, data processing, and API endpoints are handled efficiently.
API Development
CodeIgniter can be used to develop RESTful APIs that mobile apps can consume. This approach separates the backend and frontend, allowing mobile applications to fetch and send data through HTTP requests. CodeIgniter’s built-in support for RESTful services and libraries makes it a good choice for this purpose.
Setting Up CodeIgniter for Mobile App Development
Installing CodeIgniter
To begin, install CodeIgniter using Composer or by downloading the latest version from the CodeIgniter website. For this example, we’ll use Composer:
```bash composer create-project codeigniter4/appstarter myapp ```
Creating a RESTful API
Create a RESTful API in CodeIgniter to serve data to your mobile app. First, configure your database and set up your models.
Example Model: UserModel.php
```php <?php namespace App\Models; use CodeIgniter\Model; class UserModel extends Model { protected $table = 'users'; protected $primaryKey = 'id'; protected $allowedFields = ['username', 'email', 'password']; } ```
Example Controller: UserController.php
```php <?php namespace App\Controllers; use App\Models\UserModel; use CodeIgniter\RESTful\ResourceController; class UserController extends ResourceController { protected $modelName = 'App\Models\UserModel'; protected $format = 'json'; public function index() { $users = $this->model->findAll(); return $this->respond($users); } public function show($id = null) { $user = $this->model->find($id); if (!$user) { return $this->failNotFound('User not found'); } return $this->respond($user); } } ```
Consuming the API in Mobile Apps
Integrate the API with your mobile app using HTTP requests. For example, in a React Native app:
Example API Call in React Native
```javascript import React, { useState, useEffect } from 'react'; import { View, Text, Button } from 'react-native'; const App = () => { const [users, setUsers] = useState([]); useEffect(() => { fetch('http://your-api-url/users') .then(response => response.json()) .then(data => setUsers(data)); }, []); return ( <View> {users.map(user => ( <Text key={user.id}>{user.username}</Text> ))} </View> ); }; export default App; ```
Advantages of Using CodeIgniter for Native Apps
Simplified Development
By leveraging CodeIgniter for backend development, you can simplify the mobile app development process. The framework’s clear structure and extensive documentation make it easier to handle server-side logic, database interactions, and API creation.
Scalability
CodeIgniter’s lightweight nature ensures that the backend remains fast and responsive even as the app scales. This is particularly important for mobile applications, where performance can significantly impact user experience.
Conclusion
CodeIgniter’s role in mobile app development may not be immediately obvious, but its strengths as a backend framework make it a valuable tool for building native applications. By creating efficient APIs and leveraging CodeIgniter’s capabilities, developers can build powerful and scalable mobile apps.
Further Reading
Table of Contents
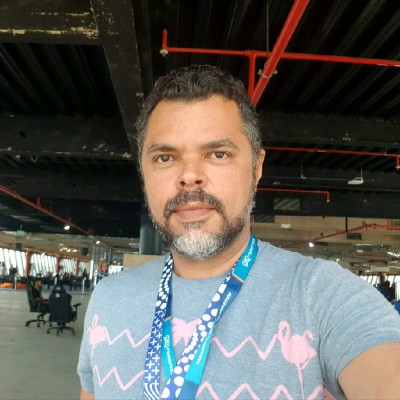
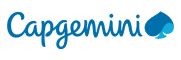