Decoding the MVC Architecture in CodeIgniter for Efficient Web Development
In the world of web development, selecting the right framework is crucial for a successful project. Among the numerous options available, CodeIgniter, a powerful PHP framework, is highly respected for its simplicity, flexibility, and efficiency. This framework has led many companies to hire CodeIgniter developers to take full advantage of its capabilities. CodeIgniter leverages the Model-View-Controller (MVC) architecture to facilitate the creation of dynamic web applications.
This article delves into CodeIgniter’s MVC structure and how it empowers web developers, particularly those skilled CodeIgniter developers you might consider for your project.
What is CodeIgniter?
CodeIgniter is an open-source PHP framework developed to simplify web application development. It offers a rich set of libraries for commonly required tasks, coupled with a straightforward interface and logical structure to access these libraries. CodeIgniter reduces the amount of code needed to develop a project, enhancing the developer’s productivity and, by extension, the project’s speed. The framework is light, compared to others in the market, further amplifying its appeal to developers who crave efficiency.
The Model-View-Controller (MVC) Architecture
The MVC architecture is a design pattern that separates an application into three interconnected components: Model, View, and Controller. This architectural pattern aims to isolate the business logic from the user interface, ensuring modularity, ease of maintenance, and scalability.
Model
The Model is responsible for handling data logic. It interacts with the database, processes data, and defines the rules for data handling and manipulation. Models are primarily concerned with the business logic of the application. They retrieve, insert, and update information in the database.
View
The View represents the user interface and everything the user interacts with. It displays data fetched from the model to the user and collects user input to pass on to the controller. Views focus on the presentation layer of the application.
Controller
The Controller acts as a coordinator between the Model and the View. It processes user input received from the View, manipulates it if necessary, interacts with the Model to perform data operations, and updates the View based on changes in the Model. The controller is essentially the glue that binds the Model and the View together.
MVC in CodeIgniter: A Deep Dive
CodeIgniter’s adoption of the MVC pattern eases the process of web development by logically separating different parts of the application.
Model in CodeIgniter
Models in CodeIgniter are PHP classes that interact with a database to fetch, insert, or update data. They contain the business logic and ‘know’ the database structure. To create a model in CodeIgniter, developers create a new file in the `application/models/` directory, where each model is a class file.
Here’s a basic example of a model:
```php class Blog_model extends CI_Model { function get_entries() { $query = $this->db->get('entries'); return $query->result(); } }
In the example, `get_entries` is a method that retrieves all rows from the ‘entries’ table in the database.
View in CodeIgniter
Views in CodeIgniter are web pages that present the user interface. They contain the HTML and form the part of the application that the user interacts with. CodeIgniter views are stored in the `application/views/` directory. Unlike Models and Controllers, Views in CodeIgniter are not classes but simple PHP files that can be loaded from a controller.
Here’s a basic example of a view:
```php <html> <head> <title>My Blog</title> </head> <body> <h1>Welcome to my Blog!</h1> <?php foreach($entries as $entry) : ?> <h2><?= $entry->title ?></h2> <p><?= $ entry->content ?></p> <?php endforeach; ?> </body> </html>
The PHP snippet within the HTML code allows the view to display dynamic data, such as blog entries.
Controller in CodeIgniter
Controllers in CodeIgniter are the heart of the application, as they control the interaction between Models and Views. Each user request goes through a Controller function, which in turn calls Model functions to get data, manipulates it if necessary, and loads the Views to present the data. Controllers in CodeIgniter are stored in the `application/controllers/` directory.
Here’s a basic example of a controller:
```php class Blog extends CI_Controller { public function index() { $this->load->model('blog_model'); $data['entries'] = $this->blog_model->get_entries(); $this->load->view('blog_view', $data); } }
In this example, the `index` method loads the ‘blog_model’, fetches all blog entries, and passes them to the ‘blog_view’.
Conclusion
CodeIgniter’s implementation of the MVC architecture plays a pivotal role in making it one of the most sought-after frameworks for PHP development. By separating the application into three interconnected components – the Model, View, and Controller – CodeIgniter enhances modularity and makes the development process highly manageable.
The simplicity and intuitiveness of the CodeIgniter framework, combined with the effectiveness of the MVC architecture, empowers developers to create efficient, scalable, and robust web applications. These characteristics make it highly beneficial to hire CodeIgniter developers for your projects. With CodeIgniter, you can build feature-rich web applications without compromising on speed and performance. It’s a powerful tool in the web developer’s arsenal, making the decision to hire CodeIgniter developers a strategic move for any organization. Understanding its MVC architecture is an investment worth making for anyone aspiring to be a successful PHP developer or for businesses seeking to enhance their web presence.
Table of Contents
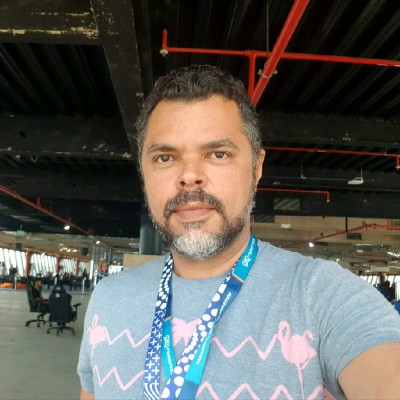
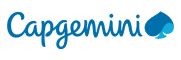