CodeIgniter and Natural Language Processing: Understanding Textual Data
Introduction to Natural Language Processing (NLP)
Natural Language Processing (NLP) is a branch of artificial intelligence that focuses on the interaction between computers and human languages. It enables machines to understand, interpret, and generate human language in a way that is both valuable and meaningful. In recent years, NLP has gained significant traction due to its applications in various fields such as sentiment analysis, chatbots, and text classification.
Integrating NLP with CodeIgniter
CodeIgniter, a popular PHP framework, is known for its simplicity and performance. While CodeIgniter itself does not have built-in NLP capabilities, it can be effectively used in conjunction with external NLP libraries and APIs to process textual data. In this blog, we will explore how to integrate NLP with CodeIgniter and demonstrate a basic implementation using Python’s NLP libraries.
Setting Up the Environment
To get started, you’ll need to set up a CodeIgniter project and prepare a Python environment for NLP. Here’s a brief overview of the steps involved:
- Install CodeIgniter: Follow the official CodeIgniter installation guide to set up a new project.
Set Up Python Environment: Install Python and necessary NLP libraries. We will use the nltk (Natural Language Toolkit) library for this example. You can install it using pip:
```bash pip install nltk ```
- Create an API for NLP: To facilitate communication between CodeIgniter and Python, we will create a simple Flask API that performs NLP tasks.
Creating the NLP API with Flask
First, install Flask if you haven’t already:
```bash pip install flask ```
Next, create a file named nlp_api.py and add the following code:
```python from flask import Flask, request, jsonify import nltk from nltk.sentiment.vader import SentimentIntensityAnalyzer app = Flask(__name__) nltk.download('vader_lexicon') sia = SentimentIntensityAnalyzer() @app.route('/analyze', methods=['POST']) def analyze(): text = request.json.get('text') if not text: return jsonify({'error': 'No text provided'}), 400 sentiment = sia.polarity_scores(text) return jsonify(sentiment) if __name__ == '__main__': app.run(debug=True) ```
This Flask application provides an endpoint /analyze that accepts POST requests with a text payload and returns sentiment analysis results.
Connecting CodeIgniter to the NLP API
In your CodeIgniter project, you will use the cURL library to communicate with the NLP API. Create a new controller, NlpController.php, and add the following code:
```php <?php defined('BASEPATH') OR exit('No direct script access allowed'); class NlpController extends CI_Controller { public function __construct() { parent::__construct(); $this->load->library('curl'); } public function analyze() { $text = $this->input->post('text'); if (empty($text)) { echo json_encode(['error' => 'No text provided']); return; } $response = $this->curl->simple_post('http://localhost:5000/analyze', json_encode(['text' => $text]), array(CURLOPT_HTTPHEADER => array('Content-Type: application/json'))); echo $response; } } ```
In this controller, we define an analyze method that sends a POST request to the Flask API with the text to be analyzed and outputs the response.
Testing the Integration
To test the integration, start the Flask server and access the analyze endpoint of your CodeIgniter application. Send a POST request with a text payload to see the sentiment analysis results.
```bash curl -X POST http://localhost/your_codeigniter_app/index.php/nlpcontroller/analyze -d "text=I love learning about NLP!" ```
You should receive a JSON response with sentiment scores.
Conclusion
Integrating NLP with CodeIgniter involves setting up an external NLP service and connecting it with CodeIgniter using API calls. This approach allows you to leverage the powerful NLP capabilities of libraries like nltk while benefiting from CodeIgniter’s robust framework features.
Further Reading
Table of Contents
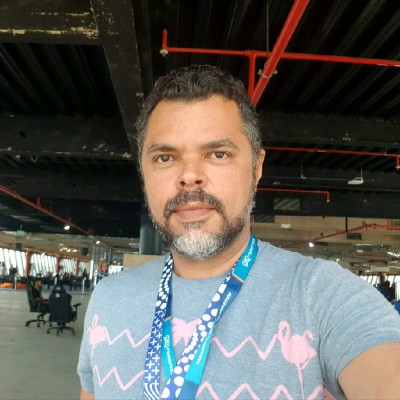
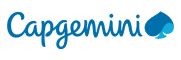