CodeIgniter and Neural Networks: Implementing Deep Learning Models
Deep learning has revolutionized many fields, from image recognition to natural language processing. Integrating these advanced models into web applications can greatly enhance their functionality. CodeIgniter, a powerful PHP framework, can serve as a robust platform for implementing neural networks and other deep learning models. This blog will guide you through the process of integrating deep learning models into a CodeIgniter application.
Setting Up the Environment
Before diving into the code, ensure you have the following tools and libraries:
- CodeIgniter: Install CodeIgniter in your project directory. You can download it from CodeIgniter’s official website.
Python and TensorFlow: Deep learning models are commonly built using Python. TensorFlow is a popular library for this purpose. Install TensorFlow with:
```bash pip install tensorflow ```
2.
3. Python Integration: Use a library like Flask or FastAPI to serve your model as an API, which can then be accessed from CodeIgniter.
Building and Serving a Neural Network Model
Let’s start by creating a simple neural network model in Python using TensorFlow.
Python Code for Deep Learning Model
Here’s a basic example of a neural network built with TensorFlow:
# Define the model model = Sequential([ Dense(64, activation='relu', input_shape=(784,)), Dense(64, activation='relu'), Dense(10, activation='softmax') ]) # Compile the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) # Train the model (for demonstration purposes) # You would typically load and preprocess your dataset here # model.fit(x_train, y_train, epochs=5) # Save the model model.save('model.h5') ```
Serving the Model with Flask
To make the model accessible from CodeIgniter, you can create a Flask API:
```python from flask import Flask, request, jsonify from tensorflow.keras.models import load_model import numpy as np app = Flask(__name__) model = load_model('model.h5') @app.route('/predict', methods=['POST']) def predict(): data = request.get_json() predictions = model.predict(np.array([data['input']])) return jsonify(predictions.tolist()) if __name__ == '__main__': app.run(port=5000) ```
Integrating with CodeIgniter
Now that we have a model and a Flask API to serve predictions, let’s integrate it with a CodeIgniter application.
CodeIgniter Controller
Create a controller to handle the communication between CodeIgniter and the Flask API:
```php <?php defined('BASEPATH') OR exit('No direct script access allowed'); class NeuralNetwork extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper('url'); } public function predict() { $input = $this->input->post('input'); $url = 'http://localhost:5000/predict'; $response = $this->make_request($url, array('input' => $input)); echo $response; } private function make_request($url, $data) { $ch = curl_init($url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data)); curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json')); $response = curl_exec($ch); curl_close($ch); return $response; } } ```
CodeIgniter View
Create a simple form to submit data to the controller:
```html <!DOCTYPE html> <html> <head> <title>Neural Network Prediction</title> </head> <body> <form action="<?php echo site_url('neuralnetwork/predict'); ?>" method="post"> <label for="input">Input:</label> <input type="text" id="input" name="input"> <button type="submit">Submit</button> </form> </body> </html> ```
Conclusion
By integrating deep learning models with CodeIgniter through a Flask API, you can harness the power of neural networks to enhance your web applications. This approach provides a scalable solution for incorporating advanced machine learning techniques into your projects.
Further Reading
Table of Contents
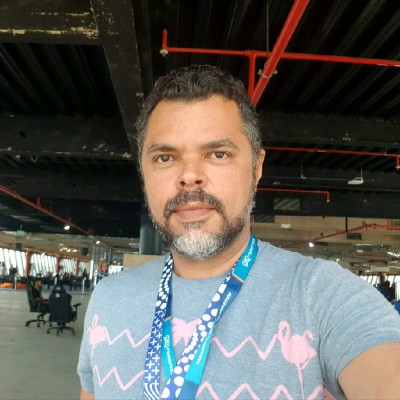
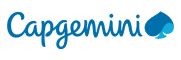