Revolutionize Your Web Development: NoSQL Integration in CodeIgniter Revealed
In the evolving landscape of web development, the need to accommodate scalability, flexibility, and dynamic data structures has never been more prevalent. Traditional relational databases often prove insufficient when dealing with massive datasets or non-relational structures, which is why NoSQL databases like MongoDB and Cassandra have gained popularity. As developers look to integrate these databases into their applications, the compatibility of frameworks becomes paramount.
CodeIgniter, a popular PHP framework, is recognized for its agility, performance, and extensive features. While it primarily supports traditional relational databases, integration with NoSQL databases is feasible and often sought-after. In this post, we’ll explore how to work with MongoDB, Cassandra, and other NoSQL databases within a CodeIgniter application.
1. MongoDB and CodeIgniter
MongoDB is a document-oriented NoSQL database, which means data is stored in JSON-like format. To work with MongoDB in CodeIgniter:
2. Setting up the library
You’ll first need a library to help you connect CodeIgniter to MongoDB. One recommended library is [Alex Bilbie’s CodeIgniter MongoDB library](https://github.com/alexbilbie/codeigniter-mongodb-library). Download and place it in your application/libraries directory.
Configuring the connection:
In the configuration file (usually application/config/mongo_db.php), adjust your settings:
```php $config['mongo_db']['active'] = 'default'; $config['mongo_db']['default']['no_auth'] = FALSE; $config['mongo_db']['default']['hostname'] = 'localhost'; $config['mongo_db']['default']['port'] = '27017'; $config['mongo_db']['default']['database'] = 'mydatabase'; $config['mongo_db']['default']['username'] = 'myusername'; $config['mongo_db']['default']['password'] = 'mypassword'; $config['mongo_db']['default']['persist'] = TRUE; $config['mongo_db']['default']['persist_key'] = 'ci_mongo_persist'; ```
Querying MongoDB:
With the library set up and configuration adjusted, querying becomes straightforward.
```php $this->load->library('mongo_db'); // Inserting a document $this->mongo_db->insert('mycollection', array('name' => 'John', 'age' => 30)); // Fetching documents $users = $this->mongo_db->where(array('age' => 30))->get('mycollection'); ```
3. Cassandra and CodeIgniter
Cassandra is a wide-column store NoSQL database. Integrating it with CodeIgniter requires a different set of tools.
4. Setting up the library
[PHPCassa](https://github.com/thobbs/phpcassa) is a popular choice for PHP. Download and include it in your application/libraries directory.
Configuring the connection:
PHPCassa usually requires a connection setup in your model or controller:
```php require_once('phpcassa/connection.php'); require_once('phpcassa/columnfamily.php'); $pool = new ConnectionPool('mykeyspace', array('localhost')); ```
Querying Cassandra:
```php $users_cf = new ColumnFamily($pool, 'users'); // Inserting data $users_cf->insert('user123', array('name' => 'John', 'age' => '30')); // Fetching data $user = $users_cf->get('user123'); ```
5. Other NoSQL Databases
While MongoDB and Cassandra are among the most popular NoSQL databases, there are others like Redis, CouchDB, and Neo4j. The integration process for these databases is analogous to the above examples:
- Find a suitable PHP library or client.
- Configure the connection in CodeIgniter.
- Query the database using the library’s functions.
For instance, with Redis, you’d use the Predis library, configure your connection, and then interact using its set of commands.
Conclusion
CodeIgniter’s extensible nature makes it possible to integrate with a myriad of NoSQL databases. While the process requires some setup, the benefits of flexibility, scalability, and high performance can make the effort worthwhile. As with any integration, understanding the specifics of the NoSQL database you’re working with, such as its data model and query language, is crucial. With the right libraries and configurations, you can empower your CodeIgniter application to leverage the full capabilities of NoSQL databases.
Table of Contents
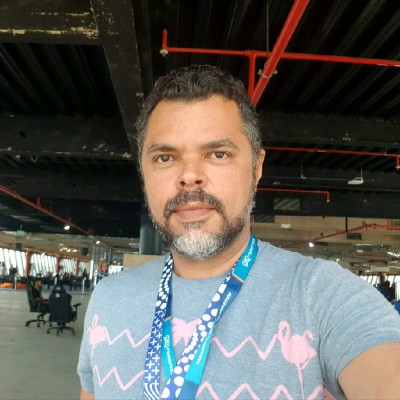
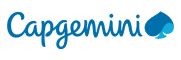