CodeIgniter and Performance Monitoring: Analyzing and Optimizing Application Speed
In today’s fast-paced digital landscape, the speed and performance of web applications are critical. Slow applications not only frustrate users but also lead to higher bounce rates and lower conversion rates. CodeIgniter, a lightweight PHP framework, offers numerous tools and techniques to monitor and optimize application performance. This blog delves into the best practices for analyzing and optimizing your CodeIgniter application’s speed, ensuring a smooth user experience.
Why Performance Monitoring Matters
Performance monitoring involves tracking and analyzing various metrics that indicate the health and efficiency of your application. These metrics include page load time, server response time, database query performance, and more. By monitoring these aspects, developers can identify bottlenecks and areas for improvement, leading to faster and more reliable applications.
Tools for Monitoring Performance in CodeIgniter
Using CodeIgniter’s Benchmarking Class
CodeIgniter comes with a built-in Benchmarking Class that helps measure the time taken to execute specific parts of your code. This is particularly useful for identifying slow-loading sections of your application.
```php $this->benchmark->mark('code_start'); // Your code here $this->benchmark->mark('code_end'); echo $this->benchmark->elapsed_time('code_start', 'code_end'); ```
In the example above, the elapsed_time function calculates the time between two markers, allowing you to pinpoint slow-performing code segments.
Database Query Profiling
CodeIgniter also provides query profiling, enabling you to see detailed information about the database queries executed during a request. This can help you identify slow or redundant queries.
To enable query profiling, set the following configuration in your database.php config file:
```php $db['default']['save_queries'] = TRUE; ```
You can then use the last_query and last_query_time methods to get details about the last executed query and its execution time.
```php echo $this->db->last_query(); echo $this->db->query_times; ```
Optimizing Application Speed
Caching
Caching is a powerful technique to reduce server load and speed up your application. CodeIgniter supports various caching mechanisms, including file-based caching and APC (Alternative PHP Cache). Implementing caching can significantly improve response times by serving static versions of your content.
Enabling Caching
To enable caching for a specific controller method, use the following code:
```php $this->output->cache(60); // Cache for 60 minutes ```
Optimizing Database Queries
Efficient database queries are crucial for maintaining fast response times. Here are a few tips:
- Use SELECT statements wisely: Avoid fetching unnecessary data. Specify only the columns you need.
- Use indexes: Index frequently used columns to speed up query execution.
- Optimize JOIN operations: Ensure that your JOINs are efficient and necessary.
Minifying Assets
Minifying CSS and JavaScript files can reduce their size, leading to faster load times. There are various tools available, such as CSSMin and JSMin, which can automate this process.
Content Delivery Network (CDN)
Using a CDN can significantly improve the loading speed of your static assets by distributing them across multiple servers worldwide. This ensures that users download assets from a server geographically closer to them.
Conclusion
Performance monitoring and optimization are ongoing processes that require continuous attention. By leveraging CodeIgniter’s built-in tools and best practices, you can ensure that your application runs efficiently and provides a seamless experience for your users. Remember, a faster application not only enhances user satisfaction but also contributes to better SEO rankings and overall success.
Further Reading
Table of Contents
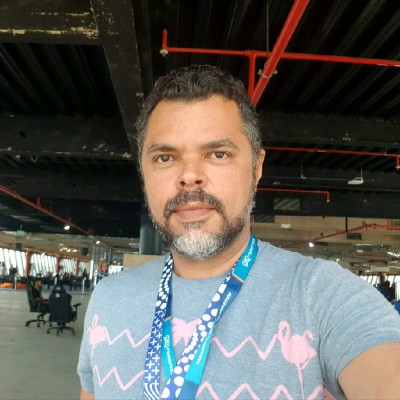
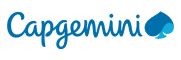