Why Your CodeIgniter App Is Slow: Performance Tips to Lead the Pack!
CodeIgniter, one of the most popular PHP frameworks, offers an elegant toolkit to build dynamic web applications. For those seeking expert guidance, hiring CodeIgniter developers can be a game-changer. However, as with any platform, achieving optimal performance is crucial for a seamless user experience. Today, we’ll delve into some effective ways to optimize your CodeIgniter application.
Table of Contents
1. Database Optimization
1.1. Efficient Database Design
Ensuring that your database is structured optimally can improve speed drastically. Normalize your database and remove any redundant data to ensure faster access.
1.2. Use Query Caching
CodeIgniter provides a query caching mechanism. By storing query results, you can avoid running the same expensive queries multiple times.
```php $this->db->cache_on(); $query = $this->db->query("YOUR QUERY HERE"); ```
Make sure to also clear cache when data changes:
```php $this->db->cache_delete('controller', 'method'); ```
1.3. Opt for Active Record Class
This class offers built-in methods to write queries, reducing the chances of errors and ensuring optimized queries.
```php $data = $this->db->select('column1, column2') ->from('table_name') ->where('column_name', $variable) ->get() ->result(); ```
2. Limiting Libraries, Helpers, and Plugins
Only load what you need. While it might seem practical to autoload libraries and helpers, this could slow down your application. Load them only when necessary.
```php $this->load->library('library_name'); $this->load->helper('helper_name'); ```
3. Caching
3.1. Full Page Caching
You can cache an entire page to reduce server stress. CodeIgniter provides an easy-to-use caching mechanism.
```php $this->output->cache($minutes); ```
3.2. OpCode Caching
Consider using OpCode caching tools like APC or OpCache. These tools cache the compiled bytecode of PHP, eliminating the need for the server to repeatedly compile PHP scripts.
4. Session Storage Management
If you’re using database sessions, ensure that the session table is indexed correctly. Regularly purge old session data. Alternatively, consider storing sessions in-memory using solutions like Redis or Memcached for faster access.
5. Minimize External Calls
Limit the number of calls to external services or APIs. When you do need them, consider using asynchronous methods or caching the results.
6. Optimizing Views and Front-end Assets
6.1. Minify JavaScript and CSS
Reduced file sizes lead to faster load times. Tools like UglifyJS or CSSNano can help.
6.2. Leverage Browser Caching
Serve assets with instructions that allow the client’s browser to cache them. This reduces redundant requests.
6.3. Implement Lazy Loading
For images and other media, consider implementing lazy loading, ensuring only visible content is loaded.
7. Use Content Delivery Network (CDN)
Deploy static assets (like images, CSS, and JavaScript files) on a CDN. CDNs distribute assets across multiple locations, serving them from the nearest node to the user.
8. Optimize Configuration Settings
8.1. Set `$config[‘compress_output’] = TRUE;
This will enable Gzip compression, reducing the size of output, leading to faster load times.
8.2. Turn Off Debugging When in Production
While debugging is essential during development, it can slow down performance in a live environment. Ensure to set `$config[‘log_threshold’] = 0;` in production.
9. Regularly Profile and Debug
CodeIgniter comes with a built-in profiler which can provide details about memory usage, execution times, and database queries.
```php $this->output->enable_profiler(TRUE); ```
Regularly check these stats to identify and rectify bottlenecks.
10. Upgrade to Latest Version
Ensure you’re always using the latest version of CodeIgniter. Updates typically come with performance enhancements and security patches.
Conclusion
Optimizing your CodeIgniter application can lead to significant speed improvements, enhancing the overall user experience. If you’re seeking professional assistance, consider hiring CodeIgniter developers for tailored solutions. The tips mentioned above are a starting point. Regular monitoring and performance testing will reveal more about your specific application’s needs. Always prioritize the end-user experience, and happy coding!
Table of Contents
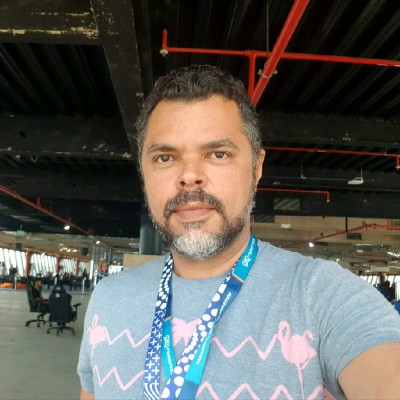
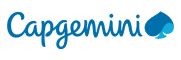