CodeIgniter and Progressive Web Apps: A Match Made for Modern Web Dev
The world of web development is always evolving. Today, users demand responsive websites with offline capabilities and real-time notifications, akin to the features they’d find in a native mobile app. Enter Progressive Web Apps (PWAs) – web applications that offer app-like experiences. When paired with a powerful PHP framework like CodeIgniter, developers can build incredibly interactive and robust web applications.
In this post, we’ll explore how to extend a CodeIgniter application with PWA features, specifically focusing on offline capabilities and push notifications.
1. What are Progressive Web Apps (PWAs)?
At their core, PWAs are web applications that use modern web capabilities to deliver app-like experiences to users. They are:
- Reliable: They load instantly and never show the “downasaur” even in uncertain network conditions.
- Fast: They respond quickly to user interactions with silky smooth animations.
- Engaging: They offer immersive full-screen experiences and can be added to the home screen, without the need for an app store.
2. Integrating PWAs with CodeIgniter
Before we begin, ensure you have a working CodeIgniter application. If you’re starting from scratch, follow the [official documentation](https://codeigniter.com/user_guide/) to set one up.
3. Offline Capabilities with Service Workers
Service Workers play a vital role in making PWAs work offline. They run in the background, independent of your web page, enabling features like content caching and push notifications.
3.1. Setting Up a Service Worker
Create a new file named `sw.js` in your root directory.
```javascript // sw.js var CACHE_NAME = 'my-cache-v1'; var urlsToCache = [ '/', '/css/styles.css', '/js/script.js' ]; self.addEventListener('install', function(event) { event.waitUntil( caches.open(CACHE_NAME) .then(function(cache) { return cache.addAll(urlsToCache); }) ); }); self.addEventListener('fetch', function(event) { event.respondWith( caches.match(event.request) .then(function(response) { if (response) { return response; } return fetch(event.request); }) ); }); ```
3.2. Registering the Service Worker
In the main HTML file of your CodeIgniter application, add the following script to register the service worker:
```javascript if ('serviceWorker' in navigator) { window.addEventListener('load', function() { navigator.serviceWorker.register('/sw.js').then(function(registration) { console.log('ServiceWorker registration successful:', registration.scope); }, function(err) { console.log('ServiceWorker registration failed:', err); }); }); } ```
With these steps, your CodeIgniter application now has offline capabilities. Users can visit your website even when they’re not connected to the internet!
4. Push Notifications
Push notifications are a great way to re-engage users. Let’s integrate them into our CodeIgniter-PWA setup.
4.1. Setting up Push Notifications
First, sign up for a service like [Firebase Cloud Messaging (FCM)](https://firebase.google.com/docs/cloud-messaging) to get your API keys.
4.2. Integrating FCM with CodeIgniter
Load the Firebase library in your CodeIgniter application. You can use Composer:
```bash composer require kreait/firebase-php ```
4.3. Sending Notifications
In your CodeIgniter controller, you can now utilize the Firebase library to send push notifications:
```php use Kreait\Firebase\Factory; use Kreait\Firebase\Messaging\CloudMessage; public function send_notification($token, $title, $body){ $factory = (new Factory)->withServiceAccount('/path/to/your/firebase/serviceAccount.json'); $messaging = $factory->createMessaging(); $message = CloudMessage::fromArray([ 'token' => $token, 'notification' => ['title' => $title, 'body' => $body], ]); $messaging->send($message); } ```
Whenever you want to send a notification, simply call the `send_notification` method with the appropriate parameters.
Wrapping Up
Pairing CodeIgniter with PWA capabilities enables developers to create robust, scalable, and interactive web applications. The offline capabilities of service workers and the engagement features of push notifications can significantly enhance user experience. It’s time to start thinking about how you can elevate your CodeIgniter projects with PWA features!
Table of Contents
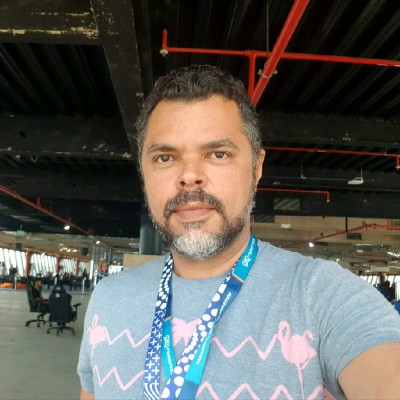
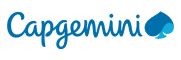