CodeIgniter Meets ReactJS: A Match Made for Single-Page Application Success!
Single-Page Applications (SPAs) have changed the landscape of modern web development. They provide a seamless user experience, with content changing dynamically without the need for a full page reload. Among the JavaScript libraries and frameworks available, ReactJS has emerged as a leader for building user interfaces in SPAs. On the backend, CodeIgniter remains a favorite lightweight PHP framework that’s both powerful and easy to use. For those looking to craft bespoke solutions, it might be beneficial to hire CodeIgniter developers who are adept at integrating with frameworks like ReactJS.
Table of Contents
In this blog post, we’ll explore how to create a SPA using CodeIgniter and ReactJS, taking advantage of the strengths of both technologies.
1. Getting Started
1.1. Setting up CodeIgniter
- Download the latest version of CodeIgniter from its official website.
- Extract the downloaded file into your web server directory.
- Configure the `base_url` and database settings in the application’s configuration files.
1.2. Setting up ReactJS
- Make sure you have Node.js and npm installed.
- Create a new React app using Create React App:
``` npx create-react-app my-app ```
- Navigate to your new app:
``` cd my-app ```
- Run the app:
``` npm start ```
Now that we have the basic setup ready, let’s get into the integration process.
2. Building the Backend with CodeIgniter
2.1. Create an API Endpoint:
We’ll build a simple API endpoint to fetch a list of items. For this, create a new controller `Api.php` in the `application/controllers` directory.
```php <?php defined('BASEPATH') OR exit('No direct script access allowed'); class Api extends CI_Controller { public function items() { $data = array( array('id' => 1, 'name' => 'Item 1'), array('id' => 2, 'name' => 'Item 2'), array('id' => 3, 'name' => 'Item 3'), ); echo json_encode($data); } } ```
2.2. Enable CORS:
To avoid CORS issues, you need to allow cross-origin requests. Edit your `.htaccess` file or server configuration to include:
``` Header set Access-Control-Allow-Origin "*" ```
3. Building the Frontend with ReactJS
3.1. Fetching Data from CodeIgniter API:
In your React app, create a new component `ItemList.js`:
```jsx import React, { useState, useEffect } from 'react'; function ItemList() { const [items, setItems] = useState([]); useEffect(() => { fetch('http://your-codeigniter-url/api/items') .then(response => response.json()) .then(data => setItems(data)); }, []); return ( <div> <h2>Item List</h2> <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); } export default ItemList; ```
3.2. Integrating the Component:
Now, integrate the `ItemList` component into your main App component.
```jsx import React from 'react'; import './App.css'; import ItemList from './ItemList'; function App() { return ( <div className="App"> <h1>CodeIgniter and ReactJS SPA</h1> <ItemList /> </div> ); } export default App; ```
Run your React app and you should see a list of items fetched from the CodeIgniter backend!
4. Enhancements & Best Practices
- State Management: For larger applications, consider using state management solutions like Redux.
- Error Handling: Implement error handling for your API requests, ensuring that user experience isn’t compromised by unforeseen issues.
- Authentication: Protect your API endpoints using JWT or other authentication methods.
- React Router: For handling multiple views and routes in your SPA, integrate React Router.
- Minify & Compress: Before deploying, ensure your JavaScript is minified and your content is gzipped for optimal performance.
Conclusion
CodeIgniter and ReactJS are powerful on their own, but when combined, they can build efficient and seamless single-page applications. For those looking to optimize this integration, it might be a wise move to hire CodeIgniter developers with expertise in such collaborations. This tutorial provided a basic merging of the two platforms, but the possibilities are truly endless.
As you build, always keep scalability in mind. Adopt best practices, stay updated with the latest releases, and above all, ensure that your user’s experience is at the heart of your development process. Happy coding!
Table of Contents
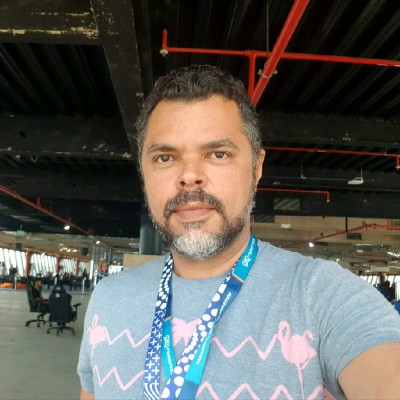
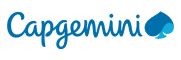