CodeIgniter Chat Mastery: Creating Dynamic Conversations with Socket.io
With the rising demand for real-time interaction on web platforms, integrating chat functionality has become a requisite feature for many web applications. While there are numerous standalone chat solutions available, integrating them seamlessly into your existing application can often be a cumbersome process.
For those utilizing CodeIgniter, a powerful PHP framework, creating a real-time chat application from scratch can be both educational and empowering. This blog post will guide you through building an instant messaging application using CodeIgniter and Socket.io.
1. Why CodeIgniter?
CodeIgniter is a robust PHP framework known for its small footprint and exceptional performance. It offers a rich set of libraries for commonly needed tasks, simple interface, and logical structure to access these libraries. Its ease of use and MVC architecture make it a popular choice among developers.
2. Why Socket.io?
Socket.io facilitates real-time communication between the server and the client. It’s a JavaScript library that enables bi-directional communication. With its event-driven architecture, you can push messages from the server to the client seamlessly, making it ideal for real-time chat applications.
Prerequisites:
- Basic knowledge of CodeIgniter.
- Node.js and NPM installed.
- CodeIgniter project set up.
3. Step-by-step Implementation
3.1. Setting Up the Chat Interface
Start by designing a simple chat interface in your CodeIgniter view. This might contain a textarea for the chat messages, an input field for typing new messages, and a button to send messages.
```html <div id="chat-box"> <div id="messages"></div> <input type="text" id="chat-input"> <button id="send-button">Send</button> </div> ```
3.2. Socket.io Setup
Install socket.io in your project:
```bash npm install socket.io ```
Create a new file for your server:
```javascript // server.js const io = require('socket.io')(3000); io.on('connection', (socket) => { console.log('New user connected'); socket.on('send-message', (message) => { io.emit('new-message', message); }); }); ```
3.3. Integrating Socket.io with CodeIgniter
On the client side, include the socket.io client script:
```html <script src="/path/to/socket.io.js"></script> ```
Then, establish a connection to the server and handle sending and receiving messages:
```javascript const socket = io.connect('http://localhost:3000'); document.getElementById('send-button').addEventListener('click', () => { const message = document.getElementById('chat-input').value; socket.emit('send-message', message); document.getElementById('chat-input').value = ''; }); socket.on('new-message', (message) => { const messagesDiv = document.getElementById('messages'); messagesDiv.innerHTML += `<p>${message}</p>`; }); ```
4. Storing Chat Messages
While real-time chat is fantastic, you might want to store messages for later retrieval. Use CodeIgniter’s model to interact with your database.
```php class Chat_model extends CI_Model { public function save_message($message) { $this->db->insert('messages', ['content' => $message]); } public function get_messages() { return $this->db->get('messages')->result(); } } ```
5. Enhancements
– User Authentication: Use CodeIgniter’s authentication library to add user login and registration. This way, you can associate messages with users.
– Private Messaging: Extend the chat functionality to support direct messages between users.
– Message Status: Implement features like ‘read receipts’ or ‘typing indicators’ using socket.io events.
Conclusion
Building a real-time chat application with CodeIgniter and Socket.io is a rewarding experience. It not only enhances user engagement on your platform but also provides invaluable insights into real-time data handling, event-driven programming, and integrating third-party libraries with CodeIgniter. With this foundation, you can further scale and enhance your chat application to cater to a broader audience and varied use cases. Happy coding!
Table of Contents
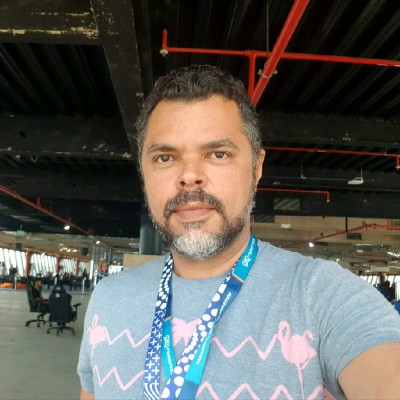
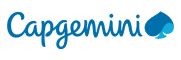