How to set up CodeIgniter for unit testing?
Setting up CodeIgniter for unit testing is a crucial step to ensure the reliability and functionality of your application code. Unit testing allows you to systematically test individual units or functions of your application in isolation to verify that they work as expected. Here’s how you can set up CodeIgniter for unit testing:
- Install a Testing Framework: CodeIgniter doesn’t include a built-in testing framework, but you can easily integrate third-party testing libraries. One popular choice is PHPUnit, a robust testing framework for PHP. You can install PHPUnit using Composer, a PHP package manager, by running:
``` composer require --dev phpunit/phpunit ```
- Create a Testing Environment: To create a separate testing environment, you should set up a new database specifically for testing. In your CodeIgniter configuration files (e.g., `database.php`), create a testing database connection with different credentials than your production database. This ensures that your tests don’t affect your live data.
- Write Test Cases: With PHPUnit installed, you can start writing test cases for your CodeIgniter application. Create test classes and methods that test specific components or functions of your application. For example, you can create a test case to check whether a model method retrieves data correctly from the database.
- Load CodeIgniter Libraries: In your test cases, you need to load CodeIgniter’s core classes and libraries to mimic the application’s environment. Use CodeIgniter’s `setUp` and `tearDown` methods to initialize and clean up resources for each test.
```php class MyModelTest extends PHPUnit_Framework_TestCase { public function setUp() { // Load CodeIgniter core classes and libraries here $this->CI =& get_instance(); $this->CI->load->library('unit_test'); $this->CI->load->model('my_model'); } public function tearDown() { // Clean up resources here } public function testGetDataFromDatabase() { // Write your test logic here $data = $this->CI->my_model->getData(); $this->assertNotEmpty($data); } } ```
- Run Tests: You can execute your unit tests by running PHPUnit from the command line:
``` vendor/bin/phpunit ```
PHPUnit will discover and run all the test cases you’ve created.
- Analyze Test Results: PHPUnit will provide you with detailed test results, including which tests passed and which failed. If any tests fail, you’ll need to investigate and fix the issues in your code.
Setting up CodeIgniter for unit testing helps you catch and fix bugs early in the development process, ensuring that your application functions correctly and maintains its integrity as it evolves. It also promotes code maintainability and allows for more confident refactoring and updates in the future.
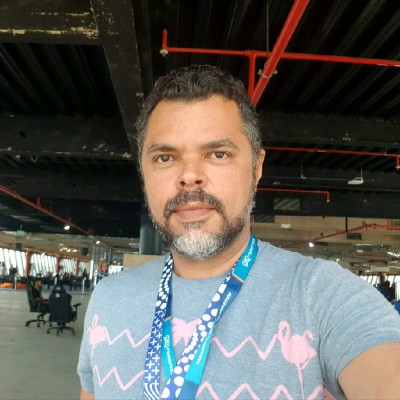
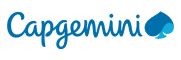