CodeIgniter Q & A
How to set up user authentication in CodeIgniter?
Setting up user authentication in CodeIgniter involves implementing a system that manages user accounts, including user registration, login, and user session management. While CodeIgniter does not provide a built-in authentication library, you can create a robust authentication system by following these steps:
- Database Setup: Start by setting up a database table to store user information, including usernames, email addresses, hashed passwords, and any additional user data you require. Use CodeIgniter’s database library to interact with the database.
- User Registration: Create a user registration form in your view that collects user details. When a user submits the form, validate the input data, hash the password using PHP’s `password_hash()` function, and insert the user’s data into the database.
- User Login: Design a login form where users can enter their credentials. Upon form submission, retrieve the user’s data from the database based on the provided username or email. Verify the password using `password_verify()` and create a user session if authentication is successful.
- Session Management: Use CodeIgniter’s session library to manage user sessions. When a user logs in, store their user ID in the session to track their authenticated status. Check the session data on protected pages to determine whether a user is logged in or not.
- Access Control: Implement access control mechanisms to restrict certain parts of your application to authenticated users. You can use CodeIgniter’s built-in functions like `$this->session->userdata()` to check user authentication status and redirect users accordingly.
- Password Reset: Create a password reset feature that allows users to reset their passwords via email. This involves generating a unique token, sending a reset link via email, and updating the password in the database after verifying the token.
- Security: Ensure security by implementing measures such as secure password storage, email verification for registration, and protection against common web vulnerabilities like SQL injection and cross-site scripting (XSS).
- Remember Me: Optionally, you can implement a “Remember Me” feature to allow users to stay logged in across sessions using long-lived cookies.
While CodeIgniter doesn’t provide a specific authentication library, you can build a robust authentication system by following these steps and leveraging CodeIgniter’s libraries and functions for database interaction, session management, and form handling. Additionally, you may consider using third-party libraries or packages specifically designed for authentication in CodeIgniter to streamline the process further.
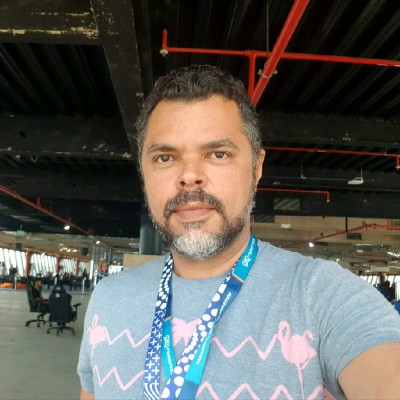
Previously at
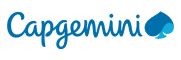
Experienced Full Stack Systems Analyst, Proficient in CodeIgniter with extensive 5+ years experience. Strong in SQL, Git, Agile.