Transform Your PHP Workflow with the Power of CodeIgniter’s Templating System
Among the plethora of open-source frameworks available for PHP development, CodeIgniter stands tall with its feature-rich toolkit and impressive performance. One of its standout features is the Templating System. In this blog post, we will delve into the nitty-gritty of using the CodeIgniter Templating System, while demonstrating how it can optimize and streamline your development process.
Understanding the Basics of CodeIgniter’s Templating System
Before we start, it’s essential to grasp what a templating system is. It is a way to segregate the logic and presentation of your application, thereby maintaining a clean and organized codebase. This separation ensures that developers can work on application logic and design independently. The main aim is to create reusable interface components, improving efficiency and reducing the possibility of errors.
CodeIgniter’s templating system, unlike others, does not force you into strict templating rules. Instead, it gives you the liberty to mold it according to your project requirements, thus facilitating your work rather than constricting it.
Getting Started
The process begins with creating a basic template, essentially a layout for your pages. This layout will contain elements common to all pages – headers, footers, navigation bars, etc. You need to leave space for the content that will change with each page.
```html <!DOCTYPE html> <html> <head> <title>{title}</title> </head> <body> <div id="header">{header}</div> <div id="content">{content}</div> <div id="footer">{footer}</div> </body> </html> ```
In the above example, the placeholders `{title}`, `{header}`, `{content}`, and `{footer}` will be replaced with actual content when rendering the page.
Building Views
In CodeIgniter, the individual components of a page are built using ‘views’. A view file is a PHP script that contains a fragment of your page. These fragments could be a header, footer, sidebar, etc.
Let’s look at how to create views for each part of the template:
- Header view (`header_view.php`):
```html <div id="logo">My Application</div> ```
- Content view (`content_view.php`):
```html <div> <h1>Welcome to My Application</h1> <p>This is my first CodeIgniter Application.</p> </div> ```
- Footer view (`footer_view.php`):
```html <div id="footer-content"> <p>© 2023 My Application</p> </div> ```
Loading the Views
Once your views are ready, you need to load them into your controller. CodeIgniter’s `load` library provides the `view` function to load a view in a controller. You need to pass the name of the view and an associative array of data you want to pass to the view.
```php public function index() { $data['title'] = 'Home'; $data['header'] = $this->load->view('header_view', '', true); $data['content'] = $this->load->view('content_view', '', true); $data['footer'] = $this->load->view('footer_view', '', true); $this->load->view('template', $data); } ```
In the above example, we’re loading the header, content, and footer views inside the `index` function of our controller. The `true` parameter tells CodeIgniter to return the view as a string, which is stored in our `$data` array. This array is then passed to our `template` view, which will replace the placeholders with the corresponding data.
The Benefits of Using CodeIgniter’s Templating System
Let’s take a closer look at how CodeIgniter’s Templating System can enhance your development experience:
- Code Reusability: Templating encourages the reuse of code. Sections like headers, footers, and sidebars, which remain constant throughout the website, can be coded once and used multiple times.
- Organized Code: Templating systems help in segregating the presentation and business logic, resulting in a clean, organized, and easy-to-read codebase.
- Parallel Development: As the view and logic are separated, developers can simultaneously work on the design and functionality, thereby enhancing the speed of the development process.
- Flexibility: CodeIgniter does not enforce a strict templating rule, providing developers the flexibility to mold the template according to their project needs.
Conclusion
Leveraging the power of CodeIgniter’s Templating System can significantly optimize your development process. It brings about better organization, encourages code reusability, and ensures the maintainability of your application. So, whether you’re a seasoned PHP developer or just starting, CodeIgniter’s Templating System offers a path to a more streamlined and efficient workflow.
Table of Contents
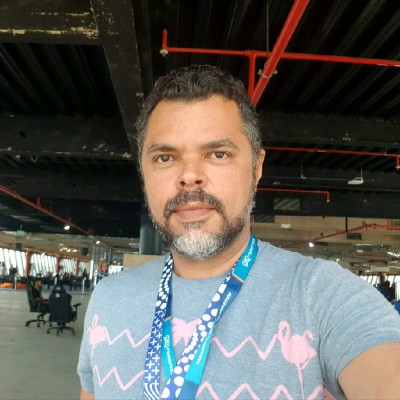
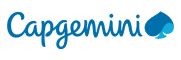