Discover How Top Developers Optimize URL Routing in CodeIgniter
CodeIgniter is one of the most popular PHP frameworks, offering developers a straightforward and elegant toolkit to create full-featured web applications. If you’re aiming to harness its full potential, you might want to hire CodeIgniter developers for specialized tasks. Among its many features, the URL routing system stands out, enabling developers to craft clean and search-friendly URLs.
Table of Contents
Let’s delve into some advanced URL routing techniques in CodeIgniter, with examples to illustrate the concepts.
1. Introduction to CodeIgniter’s URL Routing
By default, URLs in CodeIgniter follow a simple pattern:
`example.com/controller/method/parameter1/parameter2`
However, there might be scenarios where we want to modify this default structure for better URL semantics or SEO optimization. CodeIgniter’s routing capabilities come in handy in such cases.
2. Basic URL Routing
In `application/config/routes.php`, you can define custom routes:
```php $route['default_controller'] = 'welcome'; ```
The above line defines that by default, the ‘welcome‘ controller should handle requests.
3. Customizing URL Structures
Let’s imagine we have a ‘product’ controller and a method ‘details‘ that takes an ID as a parameter. A typical URL might look like:
`example.com/product/details/15`
What if we want the URL to be more descriptive? Using routing, we can achieve:
`example.com/product/15/super-cool-gadget`
The routing rule for this can be:
```php $route['product/(:num)/(:any)'] = 'product/details/\'; ```
Here, `(:num)` matches any number and `(:any)` matches any string. The `$1` in the rule refers to the first match (in this case, the product ID).
4. Routing to Specific Controllers and Methods
Suppose we want to route all URLs that start with ‘admin’ to a controller called ‘dashboard’.
```php $route['admin'] = 'dashboard'; $route['admin/(:any)'] = 'dashboard/\'; ```
Now, `example.com/admin/users` will call the ‘users’ method in the ‘dashboard’ controller.
5. Handling 404 Errors
To manage custom 404 errors:
```php $route['404_override'] = 'custom404'; ```
Here, `custom404` is a controller that handles 404 errors. Any undefined route will be managed by this controller.
6. Using Regular Expressions
CodeIgniter also supports the use of regular expressions in routing:
```php $route['products/([a-z]+)/(\d+)'] = 'products/view/\/\'; ```
This will route any URL with the format `products/some-text/some-number` to the ‘view’ method of the ‘products’ controller.
7. Redirecting and Remapping
Sometimes, you may want to remap a method request to another method within a controller. This can be done using the `_remap()` method:
```php public function _remap($method, $params = array()) { if ($method === 'old_method_name') { return $this->new_method_name($params); } show_404(); } ```
8. HTTP Verbs in Routing
CodeIgniter supports routing based on HTTP verbs, such as GET, POST, PUT, DELETE:
```php $route['product']['post'] = 'product/create'; $route['product/(:num)']['put'] = 'product/update/\'; $route['product/(:num)']['delete'] = 'product/delete/\'; ```
This way, different actions can be performed based on the HTTP verb used.
9. Handling Multiple Parameters
While you can capture multiple segments with routing, processing them might require some extra work. Consider:
```php $route['product/(:num)/(:any)/(:num)'] = 'product/details/\/\/\'; ```
Now the controller’s method will need to handle these accordingly:
```php public function details($id, $slug, $category_id) { // Your logic here } ```
10. Combining All Techniques
For maximum flexibility, consider combining different techniques:
```php $route['admin/([a-z]+)/(\d+)/edit'] = 'admin/edit_$1/\'; ```
This will route `example.com/admin/user/5/edit` to the `edit_user` method in the ‘admin’ controller and pass the ID ‘5’ as a parameter.
Conclusion
URL routing in CodeIgniter is a powerful tool that allows developers to craft tailored, clean, and search-friendly URLs. If you’re looking to enhance your projects, consider the option to hire CodeIgniter developers for expert assistance. By understanding and harnessing the advanced techniques shared above, you can offer a seamless experience for users and crawlers alike.
Remember, while customizing your routes, maintain a balance between clean URLs and the ease of understanding your routing rules. Happy coding!
Table of Contents
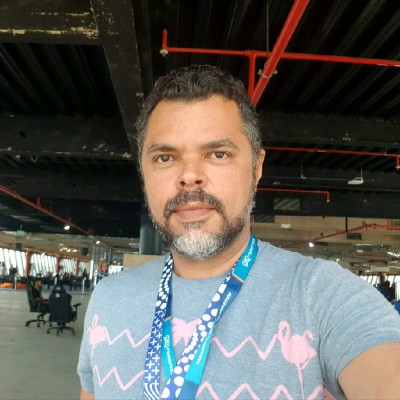
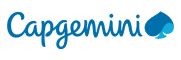