CodeIgniter and User Experience: Creating Intuitive Interfaces
User experience (UX) is pivotal in web development. A well-designed interface not only improves user satisfaction but also boosts engagement and retention. CodeIgniter, a popular PHP framework, can be a powerful tool in creating intuitive interfaces. This blog will explore how to leverage CodeIgniter for optimal user experiences, focusing on best practices and practical examples.
Understanding User Experience
What is User Experience?
User Experience refers to the overall experience a user has when interacting with a product or service, particularly in terms of ease of use, accessibility, and satisfaction. In web development, this translates to how users interact with your website or application and how effectively it meets their needs.
Importance of Intuitive Interfaces
An intuitive interface is one that feels natural and easy to use. Users should be able to navigate through your application without confusion. A well-designed interface minimizes the learning curve and reduces frustration, leading to higher user satisfaction.
CodeIgniter and User Experience
Using CodeIgniter for Intuitive Interfaces
CodeIgniter is renowned for its simplicity and flexibility. Here’s how you can use it to enhance user experience:
1. Clean and Organized Code
A clean codebase is essential for creating responsive and efficient applications. CodeIgniter’s MVC (Model-View-Controller) architecture helps in separating concerns and organizing code efficiently.
Example: Basic MVC Structure
```php // Controller: Welcome.php class Welcome extends CI_Controller { public function index() { $this->load->view('welcome_message'); } } // Model: User_model.php class User_model extends CI_Model { public function get_users() { return $this->db->get('users')->result_array(); } } // View: welcome_message.php <!DOCTYPE html> <html> <head> <title>Welcome</title> </head> <body> <h1>Welcome to CodeIgniter</h1> <p>Your application is up and running!</p> </body> </html> ```
2. Responsive Design
Incorporate responsive design to ensure your interface works well on all devices. Use CSS frameworks like Bootstrap, which integrates seamlessly with CodeIgniter.
Example: Integrating Bootstrap
Add Bootstrap to your application/views/layouts/header.php:
```html <!DOCTYPE html> <html> <head> <title>My Application</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> </head> <body> <!-- Your content here --> </body> </html> ```
3. Form Validation
Proper form validation improves user interaction by providing immediate feedback and reducing errors. CodeIgniter provides built-in form validation that you can use to enhance UX.
Example: Form Validation
```php // Controller: User.php public function register() { $this->load->library('form_validation'); $this->form_validation->set_rules('username', 'Username', 'required'); $this->form_validation->set_rules('email', 'Email', 'required|valid_email'); if ($this->form_validation->run() == FALSE) { $this->load->view('register'); } else { // Process form data } } ```
Enhancing User Experience with JavaScript
Integrating JavaScript libraries and frameworks can further improve UX. Use libraries like jQuery or frameworks like Vue.js to add dynamic elements and interactivity.
Example: Using jQuery for Enhanced UX
Add jQuery to your application/views/layouts/footer.php:
```html <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script> $(document).ready(function(){ $("button").click(function(){ alert("Button clicked!"); }); }); </script> ```
Best Practices for Intuitive Interfaces
1. Simplicity
Keep your interface simple and uncluttered. Avoid overwhelming users with too many options or complex navigation.
2. Consistency
Maintain consistency in design elements, such as buttons and colors, throughout your application to create a cohesive user experience.
3. Feedback
Provide feedback for user actions. For example, show loading indicators or success messages to keep users informed about the status of their actions.
Conclusion
Creating an intuitive user interface is crucial for delivering a positive user experience. CodeIgniter’s flexible architecture, combined with best practices in UX design, can help you build applications that are both functional and user-friendly. By following the tips outlined in this blog and leveraging CodeIgniter’s features, you can create engaging and seamless interfaces that meet users’ needs effectively.
Further Reading
Table of Contents
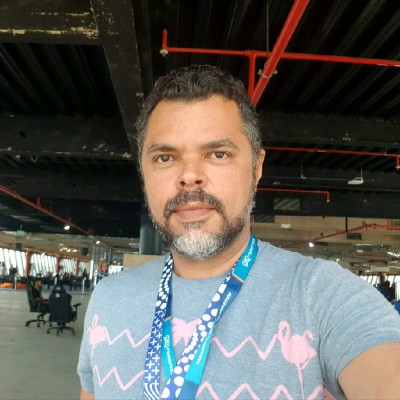
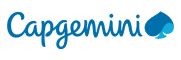