CodeIgniter and Virtual Reality: Building Immersive Web Experiences
Introduction to Virtual Reality and CodeIgniter
Virtual Reality (VR) has revolutionized the way we interact with digital content, offering immersive experiences that transport users to virtual worlds. As the demand for VR content grows, developers are exploring ways to integrate VR technologies into web applications. CodeIgniter, a powerful PHP framework, provides a solid foundation for building dynamic web applications. This blog explores how to leverage CodeIgniter to create immersive VR experiences on the web.
Setting Up the Environment
Prerequisites
Before diving into VR integration with CodeIgniter, ensure you have the following prerequisites:
- CodeIgniter Installation: Make sure you have CodeIgniter installed on your server.
- Basic Knowledge of PHP and JavaScript: Understanding these languages will be essential.
- WebVR or WebXR API: These APIs are crucial for building VR experiences in web browsers.
Installing CodeIgniter
To install CodeIgniter, follow these steps:
```bash composer create-project codeigniter4/appstarter CodeIgniter-VR cd CodeIgniter-VR php spark serve ```
This will set up a basic CodeIgniter project. Access your application at http://localhost:8080.
Integrating Virtual Reality with CodeIgniter
Choosing the Right VR Framework
To integrate VR into a web application, you need a VR framework. Popular choices include A-Frame, Three.js, and Babylon.js. For this example, we’ll use A-Frame due to its simplicity and ease of use.
Creating a Basic VR Scene
First, create a view file in CodeIgniter to render the VR scene. Let’s call it vr_view.php.
```php <!DOCTYPE html> <html> <head> <title>VR Experience</title> <script src="https://aframe.io/releases/1.2.0/aframe.min.js"></script> </head> <body> <a-scene> <a-box position="-1 0.5 -3" rotation="0 45 0" color="#4CC3D9"></a-box> <a-sphere position="0 1.25 -5" radius="1.25" color="#EF2D5E"></a-sphere> <a-cylinder position="1 0.75 -3" radius="0.5" height="1.5" color="#FFC65D"></a-cylinder> <a-plane position="0 0 -4" rotation="-90 0 0" width="4" height="4" color="#7BC8A4"></a-plane> <a-sky color="#ECECEC"></a-sky> </a-scene> </body> </html> ```
In this code snippet, we use A-Frame to define a simple VR scene with a box, sphere, cylinder, and plane. The <a-scene> tag is the main container for the VR content, and each <a-*> tag represents a 3D object.
Routing and Controller Setup
Next, set up a route and controller in CodeIgniter to display the VR scene.
1.
Create a Controller: In app/Controllers, create a file named VrController.php.
```php <?php namespace App\Controllers; class VrController extends BaseController { public function index() { return view('vr_view'); } } ```
2.
Define a Route: In app/Config/Routes.php, add the following route:
```php $routes->get('vr', 'VrController::index'); ```
Now, accessing http://localhost:8080/vr will display the VR scene.
Enhancing the VR Experience
Adding Interactivity
To make the VR experience more interactive, you can add event listeners to the objects. For example, changing the color of the box when clicked:
```html <a-box position="-1 0.5 -3" rotation="0 45 0" color="#4CC3D9" event-set__click="_event: click; material.color: red"></a-box> ```
Incorporating 360-Degree Media
To immerse users further, you can add 360-degree images or videos. Here’s an example of adding a 360-degree image as the sky:
```html <a-sky src="path_to_360_image.jpg"></a-sky> ```
Conclusion
Integrating VR into web applications using CodeIgniter and frameworks like A-Frame opens up a world of possibilities for creating immersive and interactive experiences. By leveraging the power of CodeIgniter’s MVC architecture, developers can build scalable and dynamic VR web applications. As VR technology continues to evolve, staying updated with the latest tools and techniques will be crucial for delivering cutting-edge web experiences.
Further Reading
Table of Contents
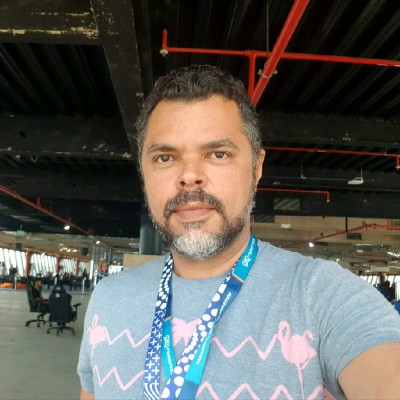
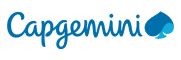