CodeIgniter and Voice Recognition: Adding Speech Capabilities to Your App
Voice recognition technology has revolutionized the way users interact with applications. By integrating voice capabilities into your CodeIgniter applications, you can create a more intuitive and accessible experience for your users. This blog will guide you through the process of adding speech recognition to your CodeIgniter app, covering the necessary steps and providing code samples for implementation.
Why Add Voice Recognition?
Voice recognition offers numerous benefits, including:
- Accessibility: It provides an alternative input method for users with disabilities.
- Efficiency: Users can interact with the app faster through voice commands.
- User Experience: It enhances the overall user experience by offering a modern and convenient interaction method.
Setting Up the Environment
Before diving into the integration, ensure that your development environment is properly set up. You will need:
- A CodeIgniter project.
- A browser that supports the Web Speech API (e.g., Google Chrome).
- Basic knowledge of JavaScript and PHP.
Implementing Voice Recognition
To implement voice recognition, we’ll use the Web Speech API, which provides easy access to speech recognition and synthesis. Here’s a step-by-step guide to adding speech capabilities to your CodeIgniter app.
1. HTML and JavaScript Setup
First, create an HTML form to capture voice input and display the transcribed text.
```html <!DOCTYPE html> <html> <head> <title>Voice Recognition in CodeIgniter</title> </head> <body> <h1>Voice Recognition Demo</h1> <button id="start-record-btn">Start Recording</button> <p id="recorded-text"></p> <script> const startRecordBtn = document.getElementById('start-record-btn'); const recordedText = document.getElementById('recorded-text'); // Check if the browser supports the Web Speech API if ('webkitSpeechRecognition' in window) { const recognition = new webkitSpeechRecognition(); recognition.continuous = false; recognition.interimResults = false; recognition.onstart = function() { console.log('Voice recognition started. Try speaking into the microphone.'); }; recognition.onresult = function(event) { const transcript = event.results[0][0].transcript; recordedText.textContent = transcript; console.log('Transcript:', transcript); }; recognition.onerror = function(event) { console.error('Error occurred in recognition:', event.error); }; startRecordBtn.addEventListener('click', () => { recognition.start(); }); } else { console.error('Web Speech API is not supported in this browser.'); } </script> </body> </html> ```
2. Processing Voice Input in CodeIgniter
After capturing the speech input, you may want to process it further in your CodeIgniter application. For example, you can save the transcribed text to a database or use it to trigger specific actions.
Controller Setup
In your SpeechController.php, you can create a function to handle the transcribed text.
```php class SpeechController extends CI_Controller { public function save_transcript() { $transcript = $this->input->post('transcript'); // Logic to save or process the transcript // For instance, saving to the database: // $this->db->insert('transcripts', ['text' => $transcript]); echo 'Transcript saved successfully!'; } } ```
JavaScript AJAX Request
Modify the JavaScript to send the transcribed text to your CodeIgniter controller.
```javascript recognition.onresult = function(event) { const transcript = event.results[0][0].transcript; recordedText.textContent = transcript; // Send the transcript to the server fetch('/speechcontroller/save_transcript', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ transcript: transcript }), }) .then(response => response.text()) .then(data => console.log(data)) .catch(error => console.error('Error:', error)); }; ```
Conclusion
Integrating voice recognition into your CodeIgniter application can significantly enhance user experience and accessibility. By leveraging the Web Speech API, you can easily add speech-to-text functionality, providing users with a modern and efficient way to interact with your app.
Further Reading
Table of Contents
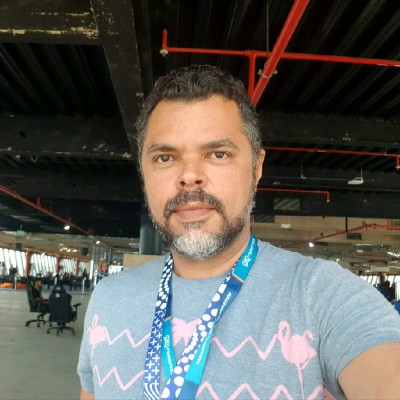
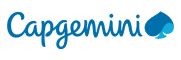