From Stripe to CRMs: Webhook Integrations in CodeIgniter Explained
The era of modern web development is all about integration. With so many services available at our fingertips, it’s essential to connect and share data efficiently. Webhooks offer an elegant solution to this challenge, providing real-time data exchange between systems.
In this blog post, we’ll explore how to integrate third-party services into a CodeIgniter application using webhooks. Let’s dive in!
1. What are Webhooks?
Webhooks are user-defined HTTP callbacks. In simple terms, they’re a way for one system to notify another system in real-time when a particular event occurs. Unlike typical API requests, which require you to poll for information, webhooks send information as it happens. It’s like a phone call rather than checking your voicemail.
2. Why Integrate Webhooks with CodeIgniter?
CodeIgniter is a popular PHP framework known for its simplicity and performance. While it offers robust features for web development, modern applications often require integration with other services, be it payment gateways, social networks, or CRM systems. Webhooks make this integration smooth and real-time.
3. Setting up a Webhook Listener in CodeIgniter
3.1. Route Configuration
Begin by setting up a route in `application/config/routes.php`:
```php $route['webhook-listener'] = 'webhook_controller/listener'; ```
3.2. Controller Setup
Next, create a controller, `Webhook_controller.php`, to handle the incoming webhook requests:
```php class Webhook_controller extends CI_Controller { public function listener() { $payload = file_get_contents('php://input'); // Process the payload $this->process_payload(json_decode($payload, true)); } private function process_payload($data) { // Handle data processing here } } ```
3.3. Payload Processing
The `process_payload` function will vary based on what you’re integrating with. For example, if you’re working with a payment gateway, you might update an order status in your database.
4. Integrating Stripe with CodeIgniter using Webhooks
Stripe is a popular payment gateway. To integrate it with CodeIgniter using webhooks:
- Set up a Webhook Endpoint on Stripe:
– Go to the Stripe Dashboard
– Navigate to Webhooks settings
– Click “Add endpoint” and add your `https://yourdomain.com/webhook-listener`.
- Modify the CodeIgniter Controller:
```php class Webhook_controller extends CI_Controller { public function listener() { $payload = file_get_contents('php://input'); $sig_header = $_SERVER['HTTP_STRIPE_SIGNATURE']; // Verify the payload with Stripe $event = null; try { $event = \Stripe\Webhook::constructEvent( $payload, $sig_header, YOUR_STRIPE_WEBHOOK_SECRET ); } catch(\UnexpectedValueException $e) { // Invalid payload http_response_code(400); exit(); } catch(\Stripe\Error\SignatureVerification $e) { // Invalid signature http_response_code(400); exit(); } // Process the Stripe event $this->process_stripe_event($event); } private function process_stripe_event($event) { switch ($event->type) { case 'payment_intent.succeeded': $paymentIntent = $event->data->object; // Update order status in your database break; // Handle other event types as needed } } } ```
- Secure the Webhook Listener:
To prevent unauthorized requests, validate the request signature using Stripe’s libraries or use a middleware approach in CodeIgniter.
5. Other Use Cases
The possibilities with webhooks are vast:
– CRM Integration: Automatically sync user data with your CRM when a user signs up.
– E-commerce: Notify users when their order status changes.
– Social Media: Share posts or updates automatically when new content is published.
Wrapping Up
Webhooks enable real-time data exchange, elevating the user experience and system efficiencies. With CodeIgniter’s flexible architecture, integrating third-party services becomes a breeze.
In this connected world, staying isolated isn’t an option. So, embrace webhooks and let your CodeIgniter application converse seamlessly with the vast universe of third-party services!
Table of Contents
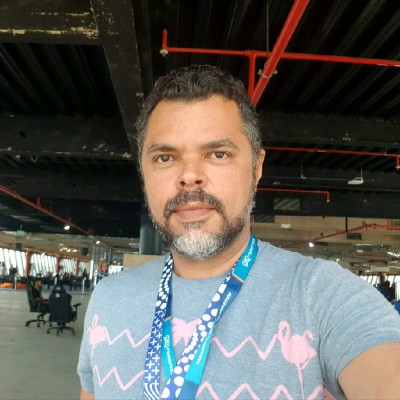
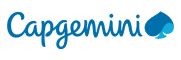