Guide to Seamless Websockets Integration in Your CodeIgniter App
Web application development has seen impressive growth over the years, leading to users expecting more dynamic, real-time interactions. To keep up with this demand, web developers are frequently exploring efficient ways to build applications with real-time capabilities.
CodeIgniter, one of the most prominent PHP frameworks, simplifies building applications with its lightweight structure and clean MVC (Model-View-Controller) architecture. While it doesn’t offer built-in support for Websockets, combining it with Websockets can create high-performing real-time applications.
In this post, we’ll walk you through setting up Websockets with CodeIgniter to achieve real-time communication in your app.
1. What are Websockets?
Websockets provide a persistent, full-duplex communication channel over a single TCP connection. Unlike traditional request-response patterns, where the client sends a request and waits for a response, Websockets allow the server to push information to the client anytime, ensuring real-time data delivery.
2. Setting up Websockets with CodeIgniter
To implement Websockets with CodeIgniter, we’ll use the Ratchet PHP library.
Step 1: Install Ratchet and Set up CodeIgniter
- First, make sure you have a running CodeIgniter application. If not, follow the [official guide](https://codeigniter.com/user_guide/installation/installing_new.html) to set one up.
- Navigate to your project directory and install Ratchet via Composer:
``` composer require cboden/ratchet ```
Step 2: Create a WebSocket Server
- In your project root, create a folder named `websockets`. Inside, create a PHP file named `server.php`.
- Add the following code to `server.php`:
```php <?php require dirname(__DIR__) . '/vendor/autoload.php'; use Ratchet\Server\IoServer; use Ratchet\Http\HttpServer; use Ratchet\WebSocket\WsServer; use MyApp\Chat; $server = IoServer::factory( new HttpServer( new WsServer( new Chat() ) ), 8080 ); $server->run(); ```
- Next, create the `MyApp` namespace by adding a folder named `MyApp` inside the `websockets` folder. Inside `MyApp`, create a file named `Chat.php` and add:
```php <?php namespace MyApp; use Ratchet\MessageComponentInterface; use Ratchet\ConnectionInterface; class Chat implements MessageComponentInterface { protected $clients; public function __construct() { $this->clients = new \SplObjectStorage; } public function onOpen(ConnectionInterface $conn) { // Store the new connection $this->clients->attach($conn); } public function onClose(ConnectionInterface $conn) { // Detach the connection when closed $this->clients->detach($conn); } public function onMessage(ConnectionInterface $from, $msg) { // Broadcast the message to all clients foreach ($this->clients as $client) { $client->send($msg); } } public function onError(ConnectionInterface $conn, \Exception $e) { $conn->close(); } } ```
Step 3: Implement Websockets in the CodeIgniter App
- In the view where you wish to implement the Websocket client, add the following JavaScript:
```javascript // Establish a connection var conn = new WebSocket('ws://your_domain_or_IP:8080'); conn.onopen = function(e) { console.log("Connection established!"); }; conn.onmessage = function(e) { // Handle the received message console.log(e.data); }; // Sending a message conn.send('Hello Server!'); ```
- Update the connection URL (`your_domain_or_IP`) with your server’s domain or IP.
Conclusion
Integrating Websockets with CodeIgniter adds a layer of dynamism to your applications, enabling real-time communication and elevating user experience. By leveraging the Ratchet PHP library, you can effortlessly implement Websockets in your CodeIgniter apps.
Remember to secure your Websockets and handle edge cases to ensure robustness. Happy coding!
Table of Contents
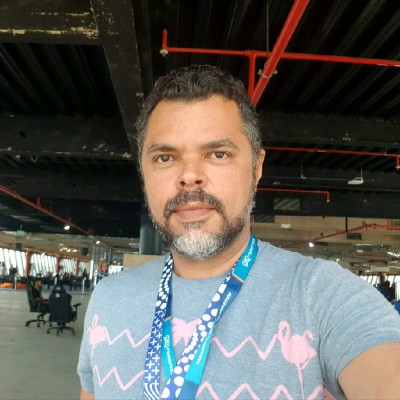
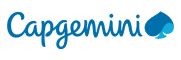