Web Accessibility Meets Django: A Guide to Inclusive Design
In the vast realm of web development, Django stands as a robust framework for rapid development and pragmatic design. However, alongside creating powerful web applications, it is crucial that we design for accessibility, ensuring that all users, regardless of physical or cognitive abilities, can interact with our applications seamlessly. The aim should always be to create inclusive web applications.
Table of Contents
Django, by design, provides developers with a toolbox of elements that can be enhanced to ensure accessibility. Let’s delve into some ways we can ensure that our Django applications are both powerful and inclusive.
1. Why is Accessibility Important?
Accessibility ensures that every user can access content, functionality, and benefits of a web application, regardless of their abilities. It’s not just a technical standard but a matter of inclusivity and equality. If a website is not accessible, it means a part of the population is being inadvertently excluded.
2. Django and Web Accessibility
Django, while being a back-end framework, inherently supports many practices that can be leveraged to produce accessible content. However, ensuring accessibility mainly relies on front-end practices. That said, Django can still be harnessed to propagate and encourage accessible practices.
2.1. Forms and Accessibility
Django’s forms are a powerful tool that developers utilize daily. With a few tweaks, these can be optimized for accessibility.
Example: Always use the `label` attribute with form fields:
```python from django import forms class CommentForm(forms.Form): name = forms.CharField(label="Your Name", max_length=100) comment = forms.CharField(label="Your Comment", widget=forms.Textarea) ```
Using `label` ensures that screen readers can read the form fields correctly. This is just the tip of the iceberg; Django forms support various attributes like `help_text` and `error_messages` that can be used to further improve accessibility.
2.2. Semantic HTML
While Django does not directly deal with HTML, its template engine encourages the use of semantic HTML.
Example: Use Django’s block tags to ensure structured content:
```html {% block header %} <header> <h1>Site Title</h1> </header> {% endblock %} {% block content %} <main> <!-- Content goes here --> </main> {% endblock %} {% block footer %} <footer> <!-- Footer content --> </footer> {% endblock %} ```
Using proper HTML elements (`<header>`, `<main>`, and `<footer>`) not only improves the page structure for screen readers but also ensures a consistent layout.
3. ARIA Attributes and Roles
While ARIA (Accessible Rich Internet Applications) is a front-end feature, Django templates can be designed to include and modify ARIA attributes dynamically.
Example: Using ARIA with Django template logic:
```html {% if errors %} <div role="alert" aria-live="assertive"> {{ errors }} </div> {% endif %} ```
By adding the ARIA attributes `role` and `aria-live`, we can direct assistive technologies to treat this div as an alert and announce its content when it changes.
4. Django Models and Alt Text
For web content like images, alt text is critical for accessibility. Django models can be structured to store and enforce the presence of alt text.
Example:
```python from django.db import models class Image(models.Model): image = models.ImageField(upload_to='images/') alt_text = models.CharField(max_length=255, blank=False) ```
By making the `alt_text` field mandatory, developers are prompted to always include descriptive text for images, aiding screen readers.
5. Accessible Pagination
Django’s paginator is another area where accessibility can be enhanced.
Example:
When displaying paginated content, use semantic elements and ARIA roles:
```html <nav aria-label="Page navigation"> <ul class="pagination"> {% if page_obj.has_previous %} <li><a href="?page=1">First</a></li> <li><a href="?page={{ page_obj.previous_page_number }}">Previous</a></li> {% endif %} <!-- ... Current page and other pages ... --> {% if page_obj.has_next %} <li><a href="?page={{ page_obj.next_page_number }}">Next</a></li> {% endif %} </ul> </nav> ```
6. Testing for Accessibility
Ensuring accessibility isn’t just about the development phase; testing is crucial. Tools like Axe, Lighthouse, and WAVE can evaluate your Django application’s accessibility. Integrate these into your development workflow to maintain high accessibility standards.
Conclusion
Building an inclusive web application is a responsibility that every developer should prioritize. While Django is predominantly a back-end framework, it offers myriad opportunities to ensure that the applications we build cater to everyone. With a combination of Django’s features and a keen eye for front-end practices, achieving an accessible and inclusive web application becomes a gratifying journey.
Let’s strive for a web that’s open and accessible to all. Remember, when we build for everyone, we also enrich the experiences for the able-bodied. Inclusivity, after all, benefits everyone.
Table of Contents
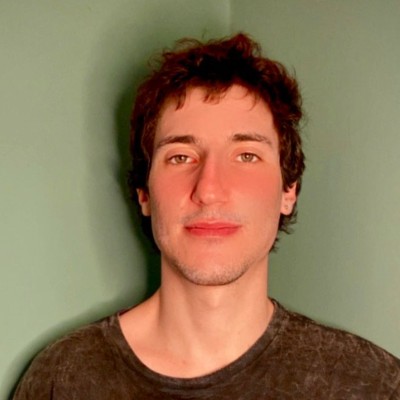
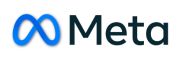