How to add search functionality to a Django website?
Adding search functionality to a Django website is a valuable feature that enhances user experience and helps users find relevant content quickly. You can implement search functionality in Django using various methods, but one of the most common and efficient ways is to use Django’s built-in search framework with a search engine like Elasticsearch or Haystack.
Here’s a step-by-step guide on how to add search functionality to a Django website:
- Install Required Packages:
Start by installing the necessary packages. For Elasticsearch-based search, you’ll need:
```bash pip install elasticsearch-dsl-django ```
If you prefer to use Haystack, install it along with a search engine backend like Whoosh, Elasticsearch, or Solr:
```bash pip install django-haystack elasticsearch ```
- Configure Your Settings:
In your Django project’s settings, configure the search engine backend and any other settings required. For Elasticsearch:
```python # settings.py HAYSTACK_CONNECTIONS = { 'default': { 'ENGINE': 'haystack.backends.elasticsearch2_backend.Elasticsearch2SearchEngine', 'URL': 'http://localhost:9200/', 'INDEX_NAME': 'myapp', }, } ```
For Whoosh:
```python # settings.py HAYSTACK_CONNECTIONS = { 'default': { 'ENGINE': 'haystack.backends.whoosh_backend.WhooshEngine', 'PATH': os.path.join(BASE_DIR, 'whoosh_index'), }, } ```
- Create Search Index:
If you’re using Haystack, create a search index for the models you want to search. If you’re using Elasticsearch, you can define your index using Elasticsearch DSL in your Django app.
- Implement Search View:
Create a view that takes user search queries, passes them to the search engine, and displays the search results.
- Create a Search Form:
Design a search form where users can enter their search queries. You can use Django’s `forms` to create a search form with input fields.
- Display Search Results:
In your search view, process user queries and retrieve search results from the search engine. Render the results in a template, making them accessible to users.
- Implement User Interface:
Design and implement a user-friendly interface for displaying search results, including pagination and sorting options.
- Testing and Optimization:
Test the search functionality thoroughly, and consider optimizing it for performance by tuning your search engine settings and using features like indexing and caching.
By following these steps, you can add robust search functionality to your Django website, making it easier for users to find the content they’re looking for. Whether you choose Elasticsearch or Haystack as your search engine, Django’s flexibility and extensibility make it a powerful choice for implementing search features tailored to your specific needs.
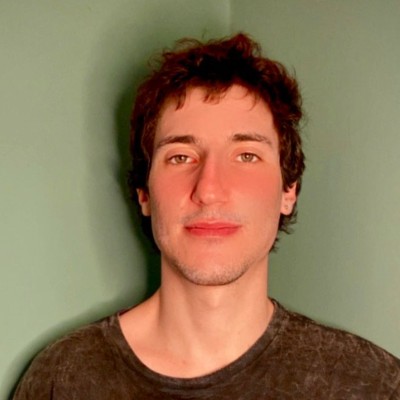
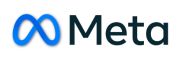