Django and Agile Development: Applying Agile Principles to Django Projects
In the fast-paced world of software development, agility is the name of the game. Agile methodology has revolutionized the way projects are managed, emphasizing collaboration, flexibility, and customer-centricity. One of the most popular web frameworks, Django, aligns seamlessly with Agile principles, allowing developers to create robust and adaptable applications. In this blog, we’ll explore how to apply Agile principles to Django projects, leveraging its features to deliver high-quality software efficiently.
Table of Contents
1. What is Agile Development?
Before diving into the synergy between Django and Agile, let’s recap what Agile development entails. Agile is a set of methodologies and practices that prioritize customer satisfaction, adaptive planning, and rapid, incremental development. It emphasizes iterative processes, continuous feedback, and close collaboration among cross-functional teams.
1.1. Agile Manifesto
The Agile Manifesto, created in 2001, lays down the foundational principles of Agile development:
- Individuals and interactions over processes and tools.
- Working software over comprehensive documentation.
- Customer collaboration over contract negotiation.
- Responding to change over following a plan.
By adhering to these principles, development teams can better respond to changing requirements, reduce development risks, and deliver valuable software sooner.
2. Django: A Framework for Agile Development
Django is a high-level Python web framework known for its simplicity, scalability, and rapid development capabilities. When used correctly, it can be a perfect companion for Agile development. Here’s how Django aligns with Agile principles:
2.1. Iterative Development
In Agile, projects progress through iterations or sprints, with each iteration delivering a potentially shippable product increment. Django’s “app” concept allows developers to modularize their codebase, making it easier to build, test, and deploy small increments of functionality. This aligns perfectly with Agile’s iterative approach.
Example: Creating a Django App
python python manage.py startapp myapp
2.2. Continuous Integration and Deployment
Agile promotes continuous integration and deployment (CI/CD) to ensure that code changes are regularly integrated into the main codebase and tested. Django supports this through various plugins and integrations, making it easy to set up automated testing and deployment pipelines.
Example: Configuring CI/CD with Django
yaml # .github/workflows/django.yml name: Django CI/CD on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Set up Python uses: actions/setup-python@v2 with: python-version: 3.x - name: Install dependencies run: | pip install -r requirements.txt pip install -r requirements-dev.txt - name: Run tests run: python manage.py test
2.3. Collaboration and Transparency
Agile teams prioritize collaboration among team members and stakeholders. Django’s built-in admin interface and REST framework allow for easy collaboration and provide transparency into the development process. Stakeholders can access and review progress, providing feedback early in the development cycle.
Example: Django Admin Interface
python # myapp/admin.py from django.contrib import admin from .models import MyModel admin.site.register(MyModel)
2.4. Flexibility to Adapt
Agile encourages teams to adapt to changing requirements, and Django’s flexibility is a huge advantage in this regard. Its robust ecosystem of packages and libraries simplifies the integration of new features and functionalities, making it easier to pivot when necessary.
Example: Adding a Package to Django
python pip install django-somepackage
2.5. Customer Feedback
Customer feedback is vital in Agile development. Django’s built-in forms and easy integration with front-end frameworks allow developers to quickly create user interfaces for collecting feedback and making improvements based on user suggestions.
Example: Django Form
python # myapp/forms.py from django import forms class FeedbackForm(forms.Form): feedback = forms.CharField(widget=forms.Textarea)
3. Applying Agile Practices to Django Projects
Now that we’ve seen how Django aligns with Agile principles, let’s explore how to apply Agile practices effectively in Django projects.
3.1. User Stories and Product Backlog
Start by defining user stories that represent features from the user’s perspective. Create a product backlog to prioritize these stories based on their importance and feasibility.
Example: User Story
Title: User Registration
Description: As a user, I want to register on the website to access exclusive content.
3.2. Sprint Planning
Plan your sprints by selecting user stories from the product backlog. Break down these stories into smaller tasks and estimate the effort required to complete each task.
Example: Sprint Planning
Sprint 1 Goals:
- User registration form
- Database model for user accounts
3.3. Daily Standup Meetings
Hold daily standup meetings to keep everyone on the same page. Discuss progress, challenges, and plans for the day. Use tools like Django’s built-in comments or Slack for remote teams.
3.4. Continuous Integration and Testing
Set up a CI/CD pipeline to automate testing and deployment. Use Django’s testing framework to write unit and integration tests for your code.
Example: Django Test
python # myapp/tests.py from django.test import TestCase from .models import MyModel class MyModelTests(TestCase): def test_my_model_creation(self): my_model = MyModel.objects.create(name="Test Model") self.assertEqual(my_model.name, "Test Model")
3.5. Iterative Development and Demo
During each sprint, focus on completing the planned tasks. At the end of the sprint, conduct a demo to showcase the completed features to stakeholders.
3.6. Retrospectives
After each sprint, conduct a retrospective meeting to review what went well, what didn’t, and how to improve the process. Use Django’s admin interface to track issues and improvements.
3.7. Product Increment and User Feedback
With each sprint, you should have a potentially shippable product increment. Gather feedback from users and stakeholders to make necessary adjustments and plan for the next sprint.
4. Tools for Agile Django Development
To streamline Agile development with Django, consider using these tools and best practices:
4.1. Version Control with Git
Use Git for version control to track changes, collaborate with the team, and manage code branches effectively.
4.2. Issue Tracking with Jira or Trello
Use tools like Jira or Trello to manage user stories, tasks, and sprint boards.
4.3. Automated Testing with pytest
While Django’s built-in testing framework is excellent, pytest offers additional testing capabilities and integrations.
4.4. Continuous Integration with Jenkins or Travis CI
Automate testing and deployment using CI/CD pipelines with Jenkins or Travis CI.
4.5. Code Review with GitLab or GitHub
Facilitate code review processes using GitLab or GitHub’s pull request features.
Conclusion
Agile development and Django are a match made in heaven for creating flexible, customer-centric web applications. By integrating Agile principles and practices into your Django projects, you can ensure that your development process remains adaptable, collaborative, and focused on delivering value to your users. Remember, the key to successful Agile development with Django is to embrace flexibility, prioritize customer feedback, and continuously improve your development process through retrospectives. So, whether you’re building a small web app or a large-scale platform, Agile and Django can help you achieve your development goals efficiently and effectively. Start applying Agile principles to your Django projects today and witness the difference in your software development process.
Table of Contents
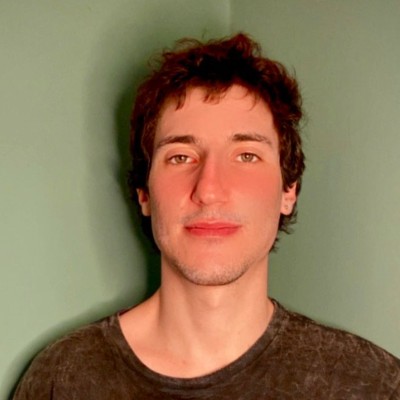
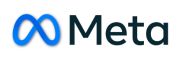