How to handle API versioning in a Django REST framework project?
Handling API versioning in a Django REST framework (DRF) project is crucial to ensure backward compatibility while evolving your API over time. Here’s how you can handle API versioning effectively:
- URL Path Versioning:
– One common approach to API versioning in DRF is using URL path versioning. In this method, you include the version number in the URL path itself. For example, you might have endpoints like `/api/v1/resource/` and `/api/v2/resource/`.
– To implement this, create separate views and serializers for each API version, and then configure your URLs to route requests to the appropriate version-specific views.
- Header Versioning:
– Another approach is to use custom headers to specify the API version. Clients can send an `Accept` or `X-API-Version` header in their requests to indicate which version of the API they want to use.
– In your DRF views, you can inspect this header and adjust the behavior accordingly.
- Accept Header Versioning:
– Some APIs use the `Accept` header to specify the version. For instance, a client can set the `Accept` header to `application/vnd.myapi.v1+json`.
– In your views, you can parse the `Accept` header to determine the requested version.
- Namespace Versioning:
– Namespace versioning involves grouping API endpoints by version within your URL configuration. For example, you might organize your URLs as `/api/v1/resource/` and `/api/v2/resource/` within separate URL namespaces.
– This approach can help keep your codebase organized as you manage multiple API versions.
- Default Versioning:
– Set a default API version to handle requests that don’t specify a version explicitly. This is especially useful when transitioning from one version to another.
– If no version is specified, you can respond with the latest stable version or provide information about available versions.
- Documentation:
– Clearly document your API versioning strategy, including how clients should specify versions in their requests. This helps developers understand and use your API effectively.
- Testing:
– Write comprehensive tests for each API version to ensure that changes do not break backward compatibility. Automated testing is crucial for maintaining stability.
- Deprecation and Sunset Policy:
– Establish a deprecation and sunset policy for older API versions. Clearly communicate to developers when older versions will no longer be supported.
- Versioning Packages:
– Consider using third-party packages like `drf-extensions` or `django-rest-framework-version-transforms` to simplify versioning implementation.
By adopting one of these versioning strategies and maintaining good documentation, testing, and communication with your API users, you can effectively manage API versioning in your Django REST framework project, ensuring a smooth evolution of your API while preserving backward compatibility for existing clients.
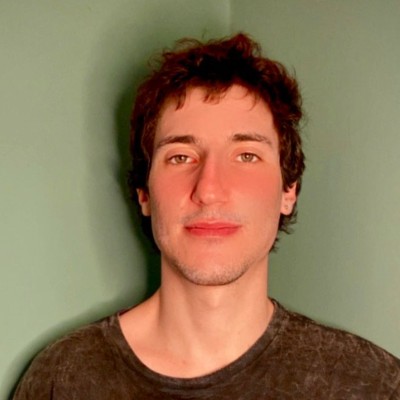
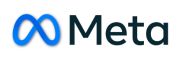