Level-Up Your Django Skills: Implement Asynchronous Task Processing with Celery
In the realm of web development, handling heavy-duty tasks can be a significant challenge, especially when maintaining a smooth user experience. With the Django framework, time-consuming tasks are most commonly executed synchronously. This means the user must wait until the task concludes, which isn’t ideal in many scenarios.
Table of Contents
Celery, an asynchronous task queue/job queue based on distributed message passing, presents a viable solution to this problem. By integrating Celery into your Django project, you can significantly enhance the user experience. This is achieved by processing tasks in the background, thereby freeing up the main thread to maintain site responsiveness. For this reason, companies often hire Django developers who are proficient in implementing Celery into their projects.
In this post, we will delve into how to integrate Celery with Django to carry out asynchronous tasks. A practical example we’ll use to illustrate this process involves sending bulk emails – a common yet potentially time-consuming task if done synchronously. As you’ll see, the capabilities Celery brings to your Django project can offer a massive boost in performance and user satisfaction.
1. Django and Celery: An Overview
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It simplifies many complex tasks in web development, including database interactions, URL routing, and form handling.
Celery is a distributed task queue for Python that can handle vast amounts of messages. It’s often used to execute background tasks or jobs that might take longer time, such as sending emails, processing images, or handling user requests on a website.
By using Celery in your Django project, you can execute time-consuming tasks asynchronously, reducing website loading time and enhancing user experience.
2. Getting Started
First, you need to install Django and Celery. Assuming you have a working Python environment, you can use pip to install these packages:
```bash pip install django pip install celery ```
Let’s create a new Django project:
```bash django-admin startproject celery_project ```
And a new Django app within the project:
```bash cd celery_project python manage.py startapp tasks_app ```
3. Setting Up Celery
Let’s set up Celery for our Django project. Create a new file in your project directory (`celery_project`) called `celery.py`:
```python # celery_project/celery.py from __future__ import absolute_import, unicode_literals import os from celery import Celery # set the default Django settings module for the 'celery' program. os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'celery_project.settings') app = Celery('celery_project') # Using a string here means the worker doesn't have to serialize # the configuration object to child processes. app.config_from_object('django.conf:settings', namespace='CELERY') # Load task modules from all registered Django app configs. app.autodiscover_tasks() ```
Also, update your Django project’s `__init__.py` file:
```python # celery_project/__init__.py from __future__ import absolute_import, unicode_literals from .celery import app as celery_app __all__ = ('celery_app',) ```
4. Implementing a Task with Celery
Now, let’s create a task to send emails. Here, we’ll simulate this with a sleep function to mimic the time-consuming process.
In your `tasks_app` directory, create a `tasks.py` file:
```python # tasks_app/tasks.py from __future__ import absolute_import, unicode_literals from celery import shared_task from time import sleep @shared_task def send_emails_task(): # Mimic long running process sleep(20) return 'Finished sending emails' ```
5. Calling Celery Task
You can call the Celery task using the `.delay()` function. Let’s create a view that triggers this task.
In your `tasks_app/views.py` file:
```python # tasks_app/views.py from django.http import HttpResponse from .tasks import send_emails_task def send_emails(request): send_emails_task.delay() return HttpResponse('Emails are being sent!') ```
And add this view to your `urls.py` file:
```python # tasks_app/urls.py from django.urls import path from .views import send_emails urlpatterns = [ path('send-emails/', send_emails, name='send-emails'), ] ```
6. Running Celery Worker
Before you can execute tasks, you need to start a Celery worker. In your command line:
```bash celery -A celery_project worker --loglevel=info ```
This will start a Celery worker with your Django project.
Conclusion
When you navigate to `http://localhost:8000/send-emails/`, the server will respond instantly, and the task of sending emails is processed in the background. This scenario demonstrates a straightforward example of how you can integrate Celery with Django to handle time-consuming tasks asynchronously. This efficiency can be a compelling reason for businesses to hire Django developers who are adept at integrating such solutions.
Do remember that in a production environment, you’ll likely want to use a more robust message broker, such as RabbitMQ or Redis. Configuring Celery to align with your project’s needs is also essential, which may include aspects like task routing, task prioritization, and failure handling.
Responsiveness is paramount for an outstanding user experience. By incorporating asynchronous task processing in your Django applications, you can ensure your users aren’t left waiting. This powerful tool, in the hands of skilled Django developers, can help create exceptionally responsive and efficient web applications.
Table of Contents
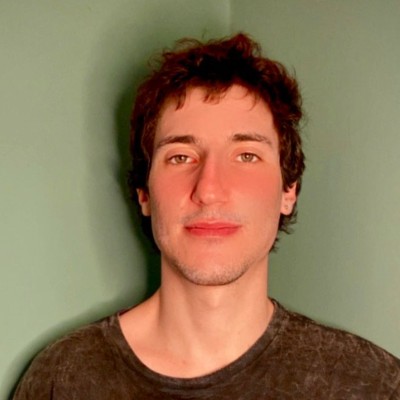
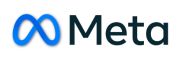