Django Meets AR: A New Frontier in Web Application Development
The incredible surge in the use of Augmented Reality (AR) technology across industries like gaming, healthcare, and e-commerce, among others, is impossible to ignore. But what if you wish to combine this cutting-edge technology with Django, a high-level web framework? This article explores how Django can be the cornerstone for AR applications, guiding you through the steps with examples to ensure you can capitalize on this potent blend.
Table of Contents
1. What is Django?
Django is a high-level, open-source web framework written in Python. It follows the “batteries-included” philosophy, which means that it provides many built-in features like authentication, database models, and an admin interface. Developers love Django because it allows them to build web applications quickly without reinventing the wheel.
2. What is Augmented Reality?
Augmented Reality (AR) is an interactive experience where objects in the real world are enhanced by computer-generated perceptual information. Unlike Virtual Reality (VR), which creates a fully artificial environment, AR overlays virtual objects on the real world.
3. How Can Django Support AR Applications?
At its core, Django handles data and serves it to clients. For AR, this means Django can manage 3D model data, user interactions, and any other relevant datasets. The client-side AR application then fetches this data, interprets it, and overlays the AR visuals on the user’s device.
4. AR Product Preview in E-commerce
4.1 Scenario
An e-commerce website selling furniture wishes to provide users with an AR experience, allowing them to visualize products in their home before purchasing.
4.2 How Django can help
- Database Storage: Using Django’s ORM, store 3D models of the furniture items, along with metadata such as dimensions, colors, etc.
- API Endpoints: Create API endpoints using Django Rest Framework (DRF) to serve these 3D models to the AR client application.
- User Interaction: Implement features where users can rate or review the AR experience or the product after visualizing it. This data can be collected and analyzed to improve user experience.
4.3 Steps
- Set up a Django model for the product:
```python class Furniture(models.Model): name = models.CharField(max_length=200) description = models.TextField() price = models.DecimalField(max_digits=6, decimal_places=2) ar_model = models.FileField(upload_to='ar_models/') dimensions = JSONField() # Storing dimensions as JSON (length, width, height) ```
- Use DRF to create an API endpoint:
```python from rest_framework import serializers, viewsets from .models import Furniture class FurnitureSerializer(serializers.ModelSerializer): class Meta: model = Furniture fields = '__all__' class FurnitureViewSet(viewsets.ModelViewSet): queryset = Furniture.objects.all() serializer_class = FurnitureSerializer ```
- In the AR client application, fetch the 3D model and overlay it in the user’s environment.
5. AR Museum Guide
5.1 Scenario
A museum wants to offer visitors an AR guide. When a visitor points their device at an exhibit, they can see historical information, reenactments, or 3D reconstructions.
5.2 How Django can help
- Content Management: The museum staff can upload and update exhibit information, videos, and 3D models through Django’s admin interface.
- Location-based Services: Implement location-awareness to serve specific content based on where the visitor is in the museum.
- Feedback Collection: After viewing an AR representation, visitors can leave feedback or ask questions through the app.
5.3 Steps
- Set up a Django model for the exhibit:
```python class Exhibit(models.Model): title = models.CharField(max_length=200) description = models.TextField() location = models.PointField() # Storing 2D coordinates ar_content = models.FileField(upload_to='ar_exhibits/') ```
- Use DRF to create an API endpoint, and integrate with a location-based service to fetch nearby exhibits.
- The AR client application fetches the relevant content when near an exhibit and displays it.
Conclusion
Django, with its robust set of features, can indeed be a game-changer in the AR domain. By managing and serving critical data to AR applications, Django ensures that developers can focus on creating immersive AR experiences without worrying about data management intricacies. By combining Django’s robustness with AR’s innovation, developers can create groundbreaking applications that enrich user experiences in countless ways.
Table of Contents
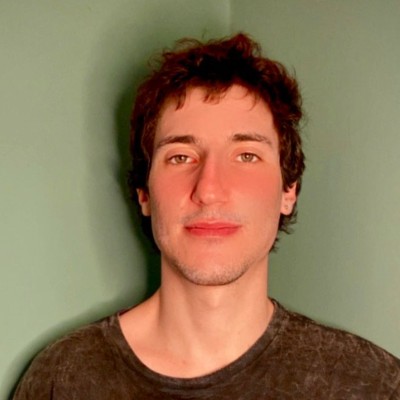
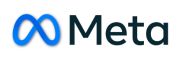