Mastering Django Automated Testing with Hands-on Examples
Django is a robust and popular web framework that allows developers to build powerful web applications quickly and efficiently. One of Django’s core tenets is the “batteries-included” philosophy, meaning it provides many tools out of the box for common development tasks. One of these tools is the built-in test framework, which can be instrumental in maintaining the quality and reliability of your web applications.
Table of Contents
In this post, we will dive into why automated testing is vital for Django applications, how to get started, and provide concrete examples to illustrate the key concepts.
1. The Importance of Automated Testing
Before we delve into the mechanics, it’s crucial to understand why automated testing is essential:
- Regression Prevention: As your application grows and evolves, testing ensures that new changes don’t break existing functionality.
- Code Confidence: When you have a robust suite of tests, developers can make changes with confidence, knowing that if something breaks, the tests will catch it.
- Documentation: Tests serve as documentation by demonstrating how the code is supposed to work.
- Quality Assurance: A comprehensive test suite reduces the chances of bugs reaching production.
2. Django’s Testing Framework
Django provides a built-in test framework that combines parts of Python’s standard `unittest` module and some additional Django-specific functionality. Here’s a quick overview of its components:
- Test Cases: Classes derived from `django.test.TestCase` that contain the actual tests.
- Test Client: A simulated browser-like client to test views and interactions.
- Fixtures: Predefined data sets that help set up the database state before tests.
- Assertions: Built-in methods to check for specific conditions or outcomes in the test cases.
3. Getting Started with Django Tests
Setting up tests in Django is straightforward. First, ensure your app structure follows the standard layout. For instance:
``` myapp/ __init__.py models.py tests.py views.py ```
Inside `tests.py`, you can start by importing necessary modules and creating your test cases.
4. A Simple Test Case Example
Imagine you have a model called `Author` in your application, defined as:
```python # models.py from django.db import models class Author(models.Model): name = models.CharField(max_length=100) bio = models.TextField() ```
You want to test the creation of an `Author` instance:
```python # tests.py from django.test import TestCase from .models import Author class AuthorTestCase(TestCase): def test_author_creation(self): author = Author.objects.create(name="Jane Doe", bio="An emerging writer.") self.assertEqual(author.name, "Jane Doe") ```
This test checks if the `Author` object is created correctly with the given name.
5. Testing Views with Django’s Test Client
Django’s Test Client allows you to simulate GET and POST requests, which is ideal for testing views. Let’s assume you have a view that lists all authors:
```python # views.py from django.shortcuts import render from .models import Author def all_authors(request): authors = Author.objects.all() return render(request, 'authors.html', {'authors': authors}) ```
You can test this view using the Test Client:
```python # tests.py from django.test import TestCase from django.urls import reverse from .models import Author class AuthorViewTestCase(TestCase): def setUp(self): self.author = Author.objects.create(name="Jane Doe", bio="An emerging writer.") def test_all_authors_view(self): response = self.client.get(reverse('all_authors')) self.assertEqual(response.status_code, 200) self.assertContains(response, "Jane Doe") ```
6. Leveraging Fixtures
For complex data setups, you can use fixtures. These are serialized data of your app’s models. To create a fixture:
```bash $ python manage.py dumpdata myapp.Author --output=myapp/fixtures/authors.json ```
To use this fixture in tests:
```python class AuthorViewTestCase(TestCase): fixtures = ['myapp/fixtures/authors.json'] # ... rest of the test case ```
This will load the data from the fixture before tests run.
Conclusion
Automated testing in Django is not just a best practice; it’s a necessity to ensure your application’s robustness and reliability. By leveraging Django’s built-in testing tools, you can maintain the quality of your web applications, reduce the potential for bugs in production, and facilitate smoother development workflows.
With the examples provided, you now have a foundational understanding of testing in Django. As your application grows, make sure to expand your test coverage and continually refine your tests to keep up with new features and changes.
Table of Contents
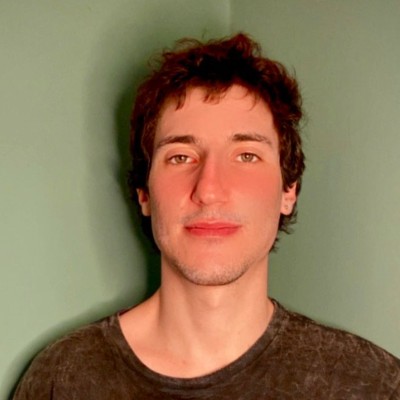
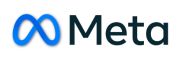