Maximize Efficiency with Django and AWS Lambda Serverless Deployments
In the current world of cloud computing, businesses constantly need to make their operations more efficient and reduce overhead costs. One of the strategies that have shown promise is serverless computing. Serverless computing is a cloud-computing execution model where the cloud provider dynamically manages the allocation and provisioning of servers.To this end, many are opting to hire Django developers, known for their expertise in one of the most efficient strategies that has shown promise – serverless computing.
Table of Contents
In the context of web application development, AWS Lambda is a noteworthy serverless computing platform. It’s one of the leading serverless platforms due to its seamless integration with other AWS services, its scalability, and the robustness it provides. This post aims to explore how to use Django, a high-level Python Web framework, with AWS Lambda for serverless computing.
1. Understanding Serverless Computing and AWS Lambda
Before we delve into the mechanics of using Django with AWS Lambda, let’s quickly go over some essential definitions. Serverless computing, in the simplest terms, allows developers to build and run applications without thinking about servers. Under the hood, there are still servers, but you do not have to provision, scale, or manage them.
AWS Lambda, Amazon’s event-driven, serverless computing platform, is an embodiment of this concept. It runs code in response to events (like changes to data in an Amazon S3 bucket or updates to a DynamoDB table) and automatically manages the computing resources required by that code.
2. Why Django?
Django, an open-source web framework written in Python, follows the Model-View-Template architectural pattern. It’s known for its simplicity and ability to help developers build complex code and applications. Django has been used in developing some of the most popular websites, including Instagram and Pinterest.
Django’s popularity stems from its ease of use, flexibility, robustness, and its extensive, rich ecosystem of middleware and pluggable apps. But most importantly, Django’s ability to work hand-in-hand with Python makes it compatible with AWS Lambda, as Python is one of the supported languages.
3. Going Serverless with Django and AWS Lambda: An Example
Let’s illustrate the process of deploying a Django application using AWS Lambda.
Before we start, ensure you have the following requirements:
- AWS Account
- Basic knowledge of Python, Django, and AWS Lambda
- Python, pip, and Django installed on your local machine
- The AWS CLI and AWS SAM CLI installed and configured
Step 1: Create a New Django Project
First, let’s create a new Django project by running the following commands:
``` $ django-admin startproject my_lambda_project $ cd my_lambda_project $ python manage.py startapp hello_world_app ```
In this case, we’ve named our Django project ‘my_lambda_project’ and created a new app within it called ‘hello_world_app‘.
Step 2: Build a Simple View
Next, let’s build a simple view in the ‘hello_world_app’ that returns a ‘Hello, World!’ message. Edit the ‘views.py’ file in your app folder:
```python from django.http import HttpResponse from django.views import View class HelloWorldView(View): def get(self, request): return HttpResponse('Hello, World!') ```
Next, let’s add this view to the URL configuration in ‘urls.py’ within the same app folder:
```python from django.urls import path from .views import HelloWorldView urlpatterns = [ path('', HelloWorldView.as_view(), name='hello_world'), ] ```
Also, ensure your new app is included in the ‘INSTALLED_APPS’ list in ‘settings.py’ and that the URLs are included in the main URL configuration.
Step 3: Prepare Django for AWS Lambda
To prepare Django for AWS Lambda, we need a WSGI (Web Server Gateway Interface) adapter. We will use ‘serverless-wsgi’. It allows AWS Lambda to communicate with our Django application.
First, install ‘serverless-wsgi’ and ‘boto3’ (AWS SDK for Python):
``` $ pip install serverless-wsgi boto3 ```
Next, we will create a ‘wsgi.py’ file in the root of our project:
```python import os import sys from django.core.wsgi import get_wsgi_application sys.path.append("/var/task") sys.path.append("/var/task/my_lambda_project") os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'my_lambda_project.settings') application = get_wsgi_application() ```
Step 4: Configure AWS SAM
AWS Serverless Application Model (SAM) is an open-source framework for building serverless applications. It provides shorthand syntax to express functions, APIs, databases, and event source mappings.
Firstly, install AWS SAM CLI:
``` $ pip install aws-sam-cli ```
Then, create a ‘template.yaml’ in the root of your project:
```yaml AWSTemplateFormatVersion: '2010-09-09' Transform: AWS::Serverless-2016-10-31 Description: Django Serverless Application Globals: Function: Timeout: 30 Resources: MyLambdaProject: Type: AWS::Serverless::Function Properties: CodeUri: . Handler: wsgi.handler Runtime: python3.8 Events: HelloWorld: Type: Api Properties: Path: /{proxy+} Method: ANY ```
Step 5: Deploy to AWS Lambda
Finally, we are ready to deploy our Django application to AWS Lambda. First, build your application:
``` $ sam build ```
Then, deploy it:
``` $ sam deploy --guided ```
After deployment, AWS will provide a URL where your application is accessible. Visiting ‘/hello_world’ on this URL will display the ‘Hello, World!’ message.
Conclusion
Deploying Django applications on AWS Lambda might appear challenging initially, but the advantages far outweigh the learning curve. Embracing serverless computing enables lower costs, greater scalability, and eliminates the need for server management. For organizations considering hiring Django developers, it is essential to realize the efficiency these professionals can bring to your operation.
As you familiarize yourself with the process, deploying Django applications on AWS Lambda becomes more intuitive, especially with the comprehensive documentation and tools provided by AWS, like the AWS SAM CLI. The shift to serverless computing empowers developers to concentrate more on application development and less on infrastructure. This refocus accelerates product delivery, cuts down costs, and boosts efficiency – a thrilling prospect in today’s cloud computing era.
With the cloud, the sky is indeed the limit – especially when you hire Django developers, whose expertise can help you fully harness this potential!
Table of Contents
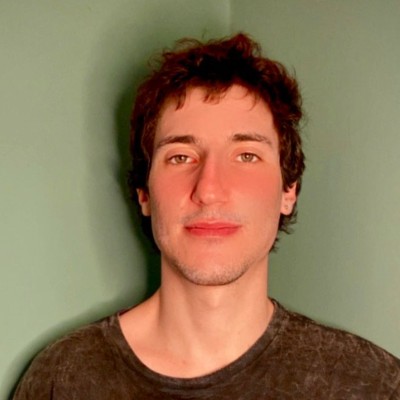
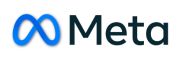