Improving Your Django Web App’s Performance with Smart Big Data Handling
Big data is omnipresent, especially in the digital age where massive amounts of data are produced every second. Regardless of the industry, dealing with large datasets has become increasingly common. In the world of web development, Django has emerged as a robust tool to address this issue. Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Its capabilities for handling big data are efficient and scalable, providing a seamless user experience even when dealing with extensive databases.
Table of Contents
This article aims to explore how Django can be utilized in handling big data and will illustrate the same through practical examples.
1. Pagination: The Essential Technique for Large Datasets
When working with large datasets, rendering all data at once can result in high server loads and slow response times, leading to a negative user experience. One common technique to mitigate these issues is pagination, which is essentially the process of dividing the data into manageable chunks or pages.
Django provides a built-in solution for this through its Paginator and Page classes. Let’s consider an example of a blog application where we have thousands of posts.
```python from django.core.paginator import Paginator from .models import Blog def blog_view(request): blog_list = Blog.objects.all() # Fetch all blog posts paginator = Paginator(blog_list, 10) # Show 10 blogs per page page = request.GET.get('page') blogs = paginator.get_page(page) return render(request, 'blog/blog_list.html', {'blogs': blogs}) ```
In the above example, we fetched all blog posts, then passed them to Django’s Paginator class along with the number of items we want to display per page. Django takes care of splitting data into multiple pages. We then fetched the current page number from the URL query parameters and used the get_page method to retrieve the blogs for that page. This data is then passed to the template for rendering.
2. Caching: An Effective Strategy for Repeated Queries
Caching is another significant technique for optimizing Django apps dealing with big data. Caching results in faster load times and better performance, as it avoids the need for Django to hit the database for repeated queries.
Django provides a robust caching framework that can cache an entire rendered HTML page or parts of it, or even the results of specific database queries. The following code caches a view for a particular duration (in seconds).
```python from django.views.decorators.cache import cache_page @cache_page(60 * 15) # Cache the view for 15 minutes def my_view(request): ... ```
Caching can also be used at a template level using the `{% cache %}` template tag. For example, if you are showing a user’s follower count on every page, it makes sense to cache this value instead of hitting the database each time.
```html {% load cache %} {% cache 5000 user_info request.user.username %} {{ request.user.profile.get_follower_count }} {% endcache %} ```
In this case, the follower count is cached for 5000 seconds for each user.
3. Database Optimizations: Select_related and Prefetch_related
Django provides several ways to optimize database queries to improve performance. Two such ways are `select_related` and `prefetch_related` methods. These methods are used to control Django’s ORM behavior when dealing with related objects.
3.1 Select_related
`select_related` is used for “one-to-many” relationships such as ForeignKey. It works by performing a SQL join and including related object data in the select statement. Here is an example:
```python def get_blog_posts(request): # Without select_related posts = BlogPost.objects.all() # Each author lookup will hit the database. # With select_related posts = BlogPost.objects.select_related('author').all() # Author data is fetched in the same query as the blog post data. ... ```
Without `select_related`, each time the author’s data is accessed, a new database query is made. By using `select_related`, the related author data is fetched in the same database query, thus reducing the total number of queries.
3.2 Prefetch_related
`prefetch_related`, on the other hand, does a separate lookup for each relationship and does “one-to-many” (“many-to-one”/”many-to-many”) relationships efficiently. It’s useful when you have a reverse foreign key relationship.
```python def get_authors(request): # Without prefetch_related authors = Author.objects.all() # Each post lookup for an author will hit the database. # With prefetch_related authors = Author.objects.prefetch_related('posts').all() # Posts data for an author is fetched in a separate query. ... ```
In the example above, without `prefetch_related`, each time the posts for an author are accessed, a new database query is made. With `prefetch_related`, the posts are fetched in a separate query, which reduces the total number of queries.
4. Asynchronous Tasks: The Power of Celery
When you need to perform heavy computations or tasks that take a significant amount of time (like sending emails, data analysis, etc.), it’s a good practice not to let your web server handle them. Otherwise, it can make your application unresponsive. That’s where asynchronous task queues come in.
Celery is a powerful, production-ready asynchronous job queue, which allows you to run time-consuming Python functions in the background. A function execution (task) can be scheduled to run asynchronously, and Django can freely respond to other user requests. Here’s an example of using Celery with Django:
First, we define a task:
```python from celery import shared_task @shared_task def add(x, y): return x + y ```
This function can be called in the view:
```python from .tasks import add def some_view(request): add.delay(4, 4) # Call the 'add' task asynchronously. ... ```
In this case, the ‘add’ function is called asynchronously using the `.delay()` method, which allows it to run in the background while Django can freely process other requests.
Conclusion
Managing big data in Django is not a daunting task, thanks to the numerous tools and techniques it provides, including pagination, caching, database optimizations, and asynchronous tasks. These techniques not only improve your app’s performance but also ensure a smoother and more enjoyable user experience. The key to dealing with big data is to identify the bottlenecks in your application and apply the right techniques that best solve those issues. Remember, every big data problem has a Django solution!
Table of Contents
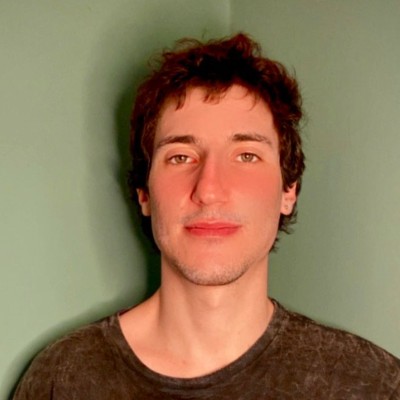
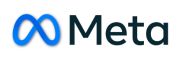