Django and Blockchain: Crafting the Future of Secure Web Apps
Django, a high-level web framework written in Python, has garnered a reputation for its simplicity and robustness. The recent surge in blockchain technology has opened up avenues to integrate blockchain features in Django applications. This article will walk you through the integration of blockchain into a Django project, providing real-world examples.
Table of Contents
1. What is Blockchain?
At its core, blockchain is a distributed, immutable ledger of transactions. Each transaction is stored in a block, and these blocks are connected chronologically to form a chain. One of the primary features of blockchain is its decentralized nature, meaning that data is stored across multiple computers or nodes.
2. Why integrate blockchain with Django?
- Transparency: By using blockchain, every transaction becomes transparent to all users, instilling trust.
- Security: Blockchain’s cryptographic nature ensures data integrity and authentication.
- Decentralization: There’s no central authority, reducing the chances of a single point of failure.
3. Implementing a simple blockchain in Django
Let’s build a basic blockchain in Django.
Step 1: Setting up a Django Project
If you haven’t set up Django, follow the official guide to create a new Django project. Once you’re set, create a new app:
```bash $ python manage.py startapp blockchain_app ```
Step 2: Defining the Blockchain
In `blockchain_app/models.py`, define a `Block` model:
```python from django.db import models import hashlib import json from time import time class Block(models.Model): index = models.IntegerField() timestamp = models.FloatField() transactions = models.TextField() proof = models.IntegerField() previous_hash = models.CharField(max_length=64) @property def hash(self): block_string = json.dumps(self.__dict__, sort_keys=True).encode() return hashlib.sha256(block_string).hexdigest() ```
Step 3: Creating the Blockchain class
In `blockchain_app/blockchain.py`:
```python class Blockchain: def __init__(self): self.chain = [] self.pending_transactions = [] # Create the genesis block self.new_block(previous_hash='1', proof=100) def new_block(self, proof, previous_hash=None): block = { 'index': len(self.chain) + 1, 'timestamp': time(), 'transactions': self.pending_transactions, 'proof': proof, 'previous_hash': previous_hash or self.hash(self.chain[-1]) } self.pending_transactions = [] self.chain.append(block) return block @staticmethod def hash(block): block_string = json.dumps(block, sort_keys=True).encode() return hashlib.sha256(block_string).hexdigest() def last_block(self): return self.chain[-1] # ... More blockchain methods, like proof_of_work, can be added here. ```
Step 4: Adding Views and Endpoints
In `blockchain_app/views.py`:
```python from django.http import JsonResponse from .blockchain import Blockchain blockchain = Blockchain() def full_chain(request): response = { 'chain': blockchain.chain, 'length': len(blockchain.chain) } return JsonResponse(response) ```
In `blockchain_app/urls.py`:
```python from django.urls import path from . import views urlpatterns = [ path('chain/', views.full_chain, name='full_chain'), ] ```
Step 5: Running the Server
Run your Django server:
```bash $ python manage.py runserver ```
Visit `http://127.0.0.1:8000/chain/` to see the full chain.
4. Advancing the Integration
The above is a rudimentary blockchain for illustrative purposes. A production-level blockchain might need:
- Consensus Algorithms: To ensure every node in the network agrees on the validity of the chain.
- Smart Contracts: To allow custom logic execution on the blockchain.
- Integration with established blockchains: Like Ethereum, to leverage their security and decentralization.
Conclusion
Incorporating blockchain with Django applications has the potential to revolutionize the way data is stored, validated, and shared. As with any integration, the effectiveness lies in the application’s use case and the need for a decentralized system. Blockchain is a powerful tool in the modern developer’s arsenal, and Django’s flexibility makes it an ideal framework for such integration.
Table of Contents
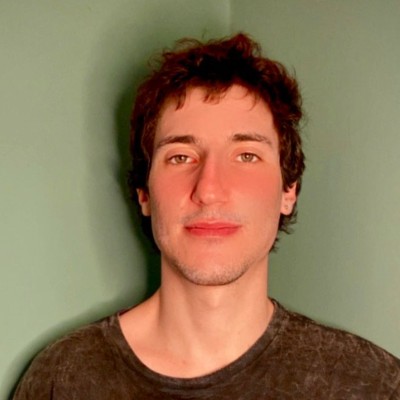
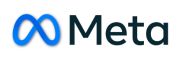