Discover How Django Is Revolutionizing the Blogging World
Django, the high-level Python web framework, is known for its robustness, scalability, and its “batteries-included” philosophy. It’s not just a web framework—it’s an ecosystem that offers developers the tools they need to build web applications, including blogging platforms. Today, we’re diving deep into how Django can be used to create a powerful blogging engine.
Table of Contents
1. Why Choose Django for a Blogging Platform?
- Mature and Stable: Django has been around for over a decade and has a proven track record.
- Scalability: As your blog grows, Django can handle increasing loads with grace.
- Security: Django provides built-in protection against many types of vulnerabilities, such as SQL injection and cross-site scripting.
- Extensibility: With Django’s app architecture, you can easily extend or customize your blogging platform.
- Community: A vast community offers tons of plugins, packages, and tutorials.
2. Example: Building a Basic Blog with Django
Here’s a step-by-step guide to creating a rudimentary blog:
2.1. Setting up Django Project:
```bash django-admin startproject myblog ```
2.2. Create the blog app:
```bash python manage.py startapp blog ```
2.3. Define your models:
In `blog/models.py`, define your `Post` model.
```python from django.db import models from django.contrib.auth.models import User class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() date_posted = models.DateTimeField(auto_now_add=True) author = models.ForeignKey(User, on_delete=models.CASCADE) ```
2.4. Admin Interface:
Django provides a built-in admin interface. Register the `Post` model in `blog/admin.py`.
```python from .models import Post admin.site.register(Post) ```
2.5. URLs and Views:
Set up your URLs in `blog/urls.py` and create corresponding views in `blog/views.py` to display blog posts.
2.6. Templates:
Design the HTML templates in `blog/templates/`.
2.7. Migrations:
Run `python manage.py makemigrations` and `python manage.py migrate` to apply the model changes to the database.
2.8. Run and Access:
```bash python manage.py runserver ```
With these basic steps, you can set up a simple blog with Django.
3. Example: Implementing a Rich Text Editor
For a blogging platform, a rich text editor can make content creation seamless. Integrating popular editors like CKEditor or TinyMCE with Django is straightforward.
3.1. Install CKEditor:
```bash pip install django-ckeditor ```
3.2. Modify models:
Replace the `content` field definition in your `Post` model:
```python from ckeditor.fields import RichTextField content = RichTextField() ```
3.3. Update settings.py:
Add `’ckeditor’,` and `’ckeditor_uploader’,` (if you’re using the image uploading feature) to the `INSTALLED_APPS`.
This enhancement will give content creators a more flexible and intuitive interface.
4. Example: Implementing User Comments
A blogging platform often thrives with user engagement, and comments are a staple.
4.1. Model for Comments:
```python class Comment(models.Model): post = models.ForeignKey(Post, related_name='comments', on_delete=models.CASCADE) author = models.ForeignKey(User, on_delete=models.CASCADE) content = models.TextField() date_posted = models.DateTimeField(auto_now_add=True) ```
4.2. Form for Comments:
Create a Django form in `blog/forms.py` for users to submit comments.
4.3. Update Views & Templates:
Extend post views and templates to handle comment creation and display.
Conclusion
With Django, the sky’s the limit. These examples are just the tip of the iceberg. You can further implement features like tags, categories, search functionality, pagination, user profiles, and much more.
Moreover, you can also leverage Django’s vast ecosystem of third-party packages to add functionalities like SEO optimization, social sharing, or analytics integration.
Building a blogging engine on Django might seem like an overkill for someone looking for a quick setup. But if you are aiming for a platform that scales, offers extensive customization, and a plethora of features, Django stands as a remarkable choice. Happy coding!
Table of Contents
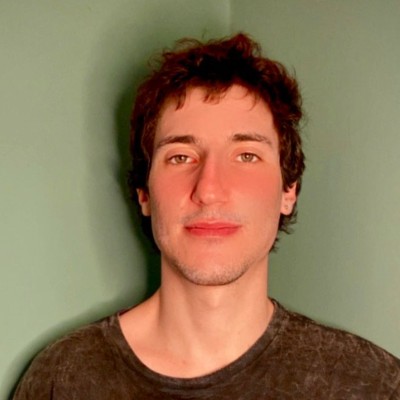
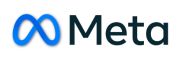