Building High-Performance APIs with Django and GraphQL: A Practical Guide
Modern web development has evolved considerably over the past decade, with numerous technologies designed to address common issues and streamline the development process. Among the most significant advancements in this area are Django and GraphQL. Django, a robust Python web framework, excels at building complex web applications with relative ease. This efficiency and ease-of-use is why many companies choose to hire Django developers for their web development needs.
Table of Contents
GraphQL, on the other hand, is a query language designed to simplify the data-fetching process. When used in conjunction with Django, these two technologies provide powerful and efficient solutions for API development. In this post, we will explore in depth the combination of Django and GraphQL, walking you through the process of creating an API using these technologies. This will illustrate why organizations opt to hire Django developers who are adept at leveraging such advanced tools to create state-of-the-art web solutions.
1. An Overview of Django and GraphQL
Django is a high-level Python Web framework that follows the model-view-template (MVT) architectural pattern. It is known for its “batteries-included” philosophy, which means it comes with a myriad of features such as an ORM (Object-Relational Mapping) system, authentication support, and more, right out of the box.
GraphQL, on the other hand, is a data query and manipulation language for APIs, and a runtime for executing those queries with your existing data. It aims to solve some of the inefficiencies of RESTful architecture by allowing the client to specify exactly what data it needs, reducing the amount of data that needs to be transferred over the network.
2. Integrating GraphQL with Django
To integrate GraphQL with Django, we’ll be using Graphene, a Python library for building GraphQL APIs in Python easily, it’s simple and optimized for Django.
2.1 Setting Up the Django Project
To start, let’s create a new Django project:
```bash pip install django django-admin startproject graphqlapi cd graphqlapi ```
Next, we create a new Django app within our project:
```bash python manage.py startapp blog ```
For our demonstration, we’re going to build a simple blog API with Posts and Authors.
In `blog/models.py`, we define our models:
```python from django.db import models class Author(models.Model): name = models.CharField(max_length=100) class Post(models.Model): title = models.CharField(max_length=100) content = models.TextField() author = models.ForeignKey(Author, on_delete=models.CASCADE) ```
Don’t forget to register these models in `blog/admin.py` and add the `blog` app to `INSTALLED_APPS` in `settings.py`.
```python # In blog/admin.py from .models import Author, Post admin.site.register(Author) admin.site.register(Post) ```
In `settings.py`:
```python INSTALLED_APPS = [ ... 'blog', ] ```
Then, run migrations:
```bash python manage.py makemigrations python manage.py migrate ```
2.2 Integrating Graphene
First, install Graphene-Django:
```bash pip install graphene-django ```
Next, update the `INSTALLED_APPS` in your `settings.py`:
```python INSTALLED_APPS = ( ... 'graphene_django', ) ```
In `settings.py`, set the `GRAPHENE` dictionary to define the schema:
```python GRAPHENE = { 'SCHEMA': 'graphqlapi.schema.schema' } ```
We need to define the GraphQL schema now. First, let’s define the types in `blog/schema.py`:
```python import graphene from graphene_django import DjangoObjectType from .models import Author, Post class AuthorType(DjangoObjectType): class Meta: model = Author fields = ("id", "name") class PostType(DjangoObjectType): class Meta: model = Post fields = ("id", "title", "content", "author") ```
Next, we define the `Query` and `Mutation` classes in the same file:
```python class Query(graphene.ObjectType): all_authors = graphene.List(AuthorType) author_by_name = graphene.Field(AuthorType, name=graphene.String(required=True)) all_posts = graphene.List(PostType) post_by_title = graphene.Field(PostType, title=graphene.String(required=True)) def resolve_all_authors(root, info): return Author.objects.all() def resolve_author_by_name(root, info, name): try: return Author.objects.get(name=name) except Author.DoesNotExist: return None def resolve_all_posts(root, info): return Post.objects.select_related("author").all() def resolve_post_by_title(root, info, title): try: return Post.objects.select_related("author").get(title=title) except Post.DoesNotExist: return None class Mutation(graphene.ObjectType): create_author = CreateAuthor.Field() create_post = CreatePost.Field() ```
The `Query` class defines the “read” operations, and the `Mutation` class handles the “write” operations.
Let’s define the `CreateAuthor` and `CreatePost` mutations:
```python class CreateAuthor(graphene.Mutation): author = graphene.Field(AuthorType) class Arguments: name = graphene.String(required=True) @staticmethod def mutate(root, info, name): author = Author(name=name) author.save() return CreateAuthor(author=author) class CreatePost(graphene.Mutation): post = graphene.Field(PostType) class Arguments: title = graphene.String(required=True) content = graphene.String(required=True) author_id = graphene.ID(required=True) @staticmethod def mutate(root, info, title, content, author_id): author = Author.objects.get(id=author_id) post = Post(title=title, content=content, author=author) post.save() return CreatePost(post=post) ```
Finally, create `schema.py` in your project directory and wire up the `Query` and `Mutation`:
```python import graphene import blog.schema class Query(blog.schema.Query, graphene.ObjectType): pass class Mutation(blog.schema.Mutation, graphene.ObjectType): pass schema = graphene.Schema(query=Query, mutation=Mutation) ```
That’s it! You’ve created a GraphQL API with Django and Graphene. You can now run the server and use a tool like GraphiQL or Apollo Client to test the API.
```bash python manage.py runserver ```
Conclusion
In this blog post, we delve into how Django and GraphQL can be used together to create efficient and powerful APIs for modern web development. This exploration showcases why many businesses choose to hire Django developers for their projects. Django, with its extensive feature set and ease of use, provides a solid foundation for web applications. It’s this robustness that makes hiring Django developers an attractive proposition.
With Django and GraphQL, you can leverage the power of Python and a strongly typed schema to build robust, scalable, and performant web applications. The marriage of these technologies is rapidly becoming a popular choice among developers for building modern web applications, thus increasing the demand to hire Django developers. If you haven’t already, give Django and GraphQL a try; you might be surprised at how much they can streamline your web development workflow.
Table of Contents
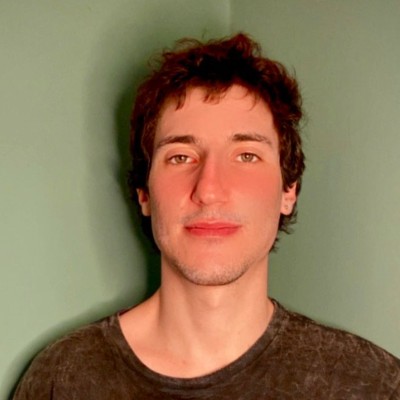
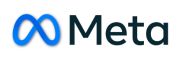