How to use Django’s built-in admin actions?
Django’s built-in admin actions are a powerful feature that allows you to perform bulk actions on selected objects in the Django admin interface. These actions can save you a significant amount of time when you need to make changes to multiple records simultaneously. Here’s how to use Django’s built-in admin actions:
- Define Custom Actions:
To use admin actions, first, define custom actions in your admin class. These actions are methods that perform specific tasks on selected objects. For example, you can create a custom action to mark selected items as “published” or “archived.”
```python from django.contrib import admin class MyModelAdmin(admin.ModelAdmin): actions = ['make_published', 'make_archived'] def make_published(self, request, queryset): queryset.update(status='published') def make_archived(self, request, queryset): queryset.update(status='archived') admin.site.register(MyModel, MyModelAdmin) ```
- Select Objects:
In the Django admin interface, navigate to the list view of the model you want to perform actions on. Select the checkboxes next to the objects you want to modify.
- Choose an Action:
From the “Action” dropdown menu at the top of the list view, select the custom action you want to perform (e.g., “Make published” or “Make archived”).
- Execute the Action:
Click the “Go” button next to the dropdown menu to execute the selected action. Django will apply the action to all the selected objects.
- Confirmation and Feedback:
Django will confirm the action and provide feedback on the number of objects affected. You’ll see a message indicating how many objects were updated.
- Customization:
You can customize the actions further by adding additional parameters or handling more complex logic within the action methods.
Django’s built-in admin actions are not limited to simple updates; you can perform various tasks, such as exporting selected items to a CSV file, sending emails, or executing any custom functionality you require. This feature significantly enhances the efficiency and usability of the Django admin interface, especially when managing large datasets or performing routine batch operations.
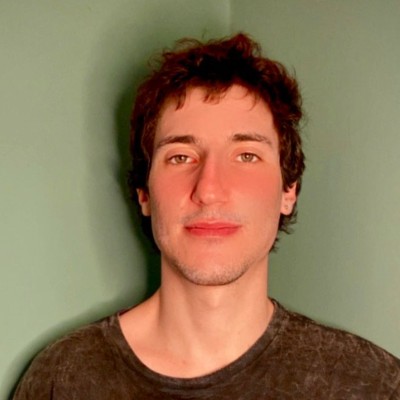
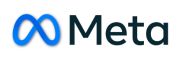