How to use built-in email sending functionality?
Django provides a straightforward and built-in way to send emails from your web application. To use Django’s built-in email sending functionality, follow these steps:
- Configure Email Settings:
In your Django project’s settings, configure the email backend and SMTP settings. You typically specify these settings in the `settings.py` file:
```python # settings.py EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend' EMAIL_HOST = 'your-smtp-server.com' EMAIL_PORT = 587 # Use the appropriate port for your SMTP server EMAIL_USE_TLS = True # Use TLS encryption (or set to False for plain SMTP) EMAIL_HOST_USER = 'your-email@example.com' # Your email address EMAIL_HOST_PASSWORD = 'your-email-password' # Your email password ```
Ensure you use the correct SMTP server settings for your email service provider.
- Import the Email Module:
In your views or wherever you want to send emails, import the `send_mail` function from `django.core.mail`:
```python from django.core.mail import send_mail ```
- Send an Email:
Use the `send_mail` function to send an email. It takes several arguments, including the subject, message, sender, recipient(s), and optional HTML message:
```python send_mail( 'Subject of the Email', 'Message of the Email', 'your-email@example.com', # Sender's email address ['recipient@example.com'], # List of recipient email addresses fail_silently=False, # Set to True to suppress exceptions on failure ) ```
You can also send HTML-formatted emails by providing an HTML message as the `message` argument and setting the content type to `’text/html’`.
- Advanced Email Sending:
Django allows you to send more complex emails with attachments, HTML templates, and inline images. You can use the `EmailMessage` class or the `send_mass_mail` function for more advanced email sending requirements.
- Error Handling:
Consider handling exceptions that may occur during email sending, such as network issues or incorrect SMTP settings. Set `fail_silently` to `True` in the `send_mail` function to suppress exceptions or implement custom error handling logic.
With these steps, you can easily leverage Django’s built-in email sending functionality to send emails from your web application. Whether it’s sending user registration confirmations, password reset links, or notifications, Django’s email capabilities provide a robust and reliable way to communicate with users and clients.
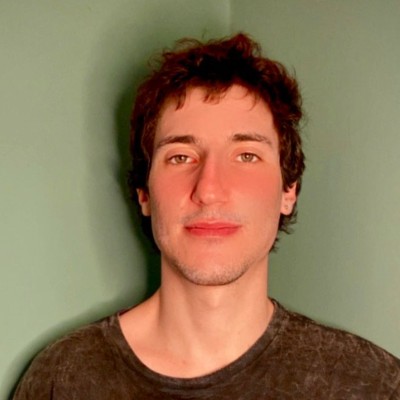
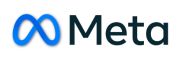