How to use built-in middleware in Django?
Django’s built-in middleware is a fundamental component of the Django framework that allows you to process requests and responses globally before they reach your view functions or after they leave them. Middleware provides a way to add cross-cutting concerns and functionalities to your web application without modifying each view individually. Here’s a guide on how to use Django’s built-in middleware effectively:
- Understanding Middleware: Middleware in Django is a series of functions or classes that Django runs in sequence for every HTTP request and response cycle. Middleware can perform various tasks such as authentication, request/response modification, security checks, and more.
- Configuration: To use built-in middleware in Django, you can add middleware classes to the `MIDDLEWARE` setting in your project’s `settings.py` file. This setting defines the order in which middleware classes are executed, from top to bottom. For example:
```python MIDDLEWARE = [ 'django.middleware.security.SecurityMiddleware', 'django.contrib.sessions.middleware.SessionMiddleware', 'django.middleware.common.CommonMiddleware', # ... ] ```
- Common Use Cases: Some common use cases for Django middleware include:
– Authentication and Authorization: Middleware can handle user authentication and permission checks globally.
– Request/Response Modification: You can modify incoming requests or outgoing responses to add headers, manipulate content, or apply URL rewriting.
– Security: Middleware can add security features like Cross-Site Request Forgery (CSRF) protection and Content Security Policy (CSP) headers.
– Caching: Implement caching mechanisms to improve performance.
– Logging: Middleware can log request/response information or application-specific data.
– Custom Functionality: You can create custom middleware to perform application-specific tasks.
- Custom Middleware: Django allows you to create custom middleware by defining your own functions or classes. To create custom middleware, define a class with methods like `__init__`, `__call__`, or `process_request` and `process_response`, depending on when you want your middleware to execute. Add your custom middleware to the `MIDDLEWARE` setting to include it in the middleware execution sequence.
- Order Matters: Be mindful of the order in which middleware classes are listed in the `MIDDLEWARE` setting, as this determines the execution sequence. Middleware classes at the beginning of the list are executed first, while those at the end are executed last.
Django’s built-in middleware is a powerful tool for adding global functionality and processing to your web application. By configuring and customizing middleware classes, you can efficiently implement a wide range of features and behaviors without cluttering your view functions with repetitive code, making your application more maintainable and modular.
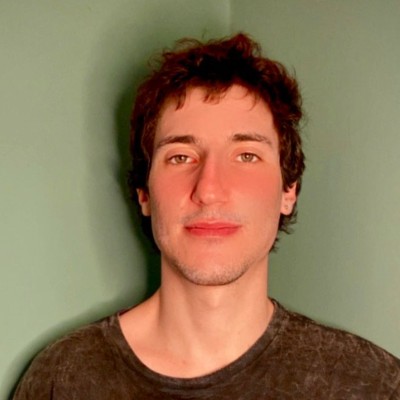
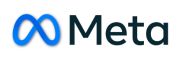