How to use built-in session management in Django?
Django’s built-in session management provides a convenient way to store and retrieve user-specific data across multiple HTTP requests. Sessions are particularly useful for maintaining user authentication, storing shopping cart contents, or any other data that needs to persist between requests. Here’s how you can use Django’s session management:
- Session Configuration:
To enable session management in Django, you need to ensure that the `django.contrib.sessions.middleware.SessionMiddleware` middleware is included in your project’s `MIDDLEWARE` setting.
```python MIDDLEWARE = [ # ... 'django.contrib.sessions.middleware.SessionMiddleware', # ... ] ```
- Session Storage:
Django allows you to choose the backend storage mechanism for sessions. You can use the default database-backed storage, or opt for alternatives like using in-memory caching (e.g., Redis or Memcached). To specify the session engine, set the `SESSION_ENGINE` and `SESSION_COOKIE_NAME` in your project’s settings:
```python SESSION_ENGINE = 'django.contrib.sessions.backends.cache' # Use caching for sessions SESSION_COOKIE_NAME = 'my_session_cookie' # Customize session cookie name ```
- Session Usage:
Once configured, you can use sessions in your views and templates. To store data in a session, use the `request.session` attribute, which behaves like a Python dictionary:
```python # Storing data in a session request.session['user_id'] = user.id # Retrieving data from a session user_id = request.session.get('user_id') ```
- Session Expiry:
By default, Django sessions expire when the user closes their browser (session cookies). You can customize the session expiration period by setting the `SESSION_COOKIE_AGE` (in seconds) in your settings:
```python SESSION_COOKIE_AGE = 3600 # Session expires after 1 hour ```
- Session Security:
Ensure that session data is protected against tampering by using Django’s built-in mechanisms. Session data is stored in a signed cookie, preventing unauthorized changes. Additionally, you can enable HTTPS to encrypt the session data during transmission.
- Session Cleanup:
Django provides management commands for cleaning up expired sessions from the database. You can run this periodically to free up database space:
```bash python manage.py clearsessions ```
- Session-Based Authentication:
You can integrate session management with Django’s built-in authentication system to keep users logged in between requests. It’s a fundamental component for creating user sessions in web applications.
Django’s built-in session management is a powerful feature that simplifies the handling of user-specific data across web requests. By configuring and using sessions effectively, you can enhance the user experience of your Django web application while maintaining security and flexibility.
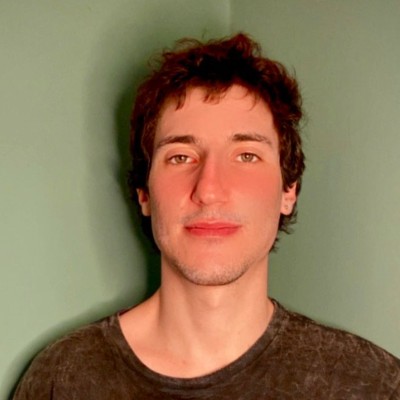
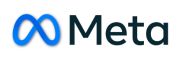