How to use built-in user authentication views in Django?
Django’s built-in user authentication views provide a convenient way to handle common authentication tasks such as user registration, login, password reset, and logout without writing extensive code from scratch. These views are part of Django’s `django.contrib.auth` module and can be easily integrated into your web application. Here’s a guide on how to use Django’s built-in user authentication views effectively:
- URL Configuration: Start by configuring the URLs for authentication views. In your project’s `urls.py`, include the authentication views by importing `auth` from `django.contrib` and adding them to your URL patterns. For example:
```python from django.contrib.auth import views as auth_views urlpatterns = [ # ... path('login/', auth_views.LoginView.as_view(), name='login'), path('logout/', auth_views.LogoutView.as_view(), name='logout'), path('password_reset/', auth_views.PasswordResetView.as_view(), name='password_reset'), path('password_reset_done/', auth_views.PasswordResetDoneView.as_view(), name='password_reset_done'), # ... ] ```
This example includes URL patterns for login, logout, password reset, and password reset confirmation views.
- Templates: Django’s authentication views come with default templates for each view. You can override these templates to match your application’s design. To do this, create template files in your app’s “registration” directory with the same names as the default templates (e.g., “login.html,” “password_reset.html”) and customize them as needed.
- Authentication Forms: Django’s authentication views use built-in authentication forms for login and password reset. You can customize these forms by creating custom forms that inherit from `AuthenticationForm` and `PasswordResetForm`. This allows you to add extra fields or modify validation behavior.
- User Redirects: You can specify where users should be redirected after successful login or logout by setting the `LOGIN_REDIRECT_URL` and `LOGOUT_REDIRECT_URL` in your project’s `settings.py` file. For example:
```python LOGIN_REDIRECT_URL = 'dashboard' LOGOUT_REDIRECT_URL = 'home' ```
This ensures that users are redirected to the appropriate pages after authentication actions.
- Custom Views and Logic: While Django’s built-in authentication views cover many common scenarios, you can extend and customize authentication logic by creating your views and using Django’s authentication functions and decorators. For example, you might want to add user registration with additional profile information.
By using Django’s built-in user authentication views and customizing them as needed, you can save development time and ensure that your web application has robust and secure authentication functionality. These views are a valuable part of Django’s authentication system, allowing you to focus on building the core features of your application.
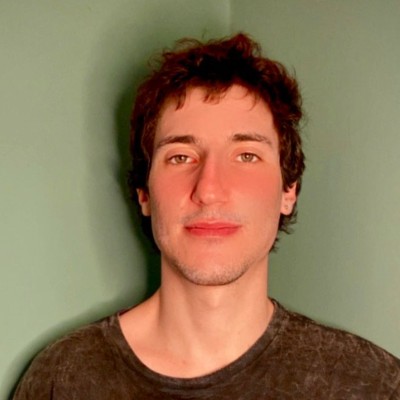
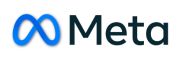