Django Q & A
How to use Django’s class-based views?
Django’s class-based views (CBVs) provide a powerful and organized way to handle HTTP requests and responses in your web applications. They offer a more reusable and structured approach compared to function-based views. Here’s how to use Django’s class-based views effectively:
- Import CBVs: Begin by importing the necessary CBVs in your views.py file. Django provides a wide range of generic CBVs, such as `DetailView`, `ListView`, `CreateView`, `UpdateView`, and `DeleteView`, which you can extend to build specific views.
- Create CBV Classes: Define your CBV classes by subclassing the appropriate generic CBV. Each CBV class typically represents a specific view in your application. For example, to create a view for displaying a list of objects, you can create a class like this:
```python from django.views.generic import ListView from .models import YourModel class YourModelListView(ListView): model = YourModel template_name = 'your_app/your_model_list.html' context_object_name = 'object_list' ```
- Define Attributes: Customize your CBV by defining attributes like `model`, `template_name`, and `context_object_name` to specify the model to work with, the template to render, and the context variable name.
- URL Configuration: Map your CBV to a URL in your project’s `urls.py` file. Use the `as_view()` method to convert the CBV class into a view function that Django can use in URL patterns.
```python from django.urls import path from .views import YourModelListView urlpatterns = [ path('your_model/', YourModelListView.as_view(), name='your_model-list'), ] ```
- Template Rendering: Create templates in your application’s template directory (as specified in `template_name`) to render the HTML content. Use the context variable (as specified in `context_object_name`) to access data in the template.
- Additional Methods: CBVs often provide additional methods and attributes for handling different HTTP methods (e.g., `get()`, `post()`), form processing, and other view-specific logic. You can override these methods to customize behavior as needed.
- Inheritance: CBVs support class inheritance, allowing you to create reusable view components and easily override or extend their functionality in child classes.
By using Django’s class-based views, you can maintain clean and organized view code, promote code reuse, and take advantage of the built-in generic views to simplify common tasks. CBVs are a powerful tool for building robust web applications in Django.
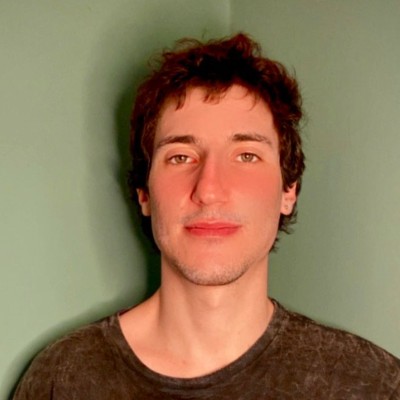
Previously at
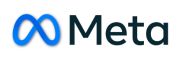
Experienced Full-stack Developer with a focus on Django, having 7 years of expertise. Worked on diverse projects, utilizing React, Python, Django, and more.