How to create custom middleware in Django?
Creating custom middleware in Django allows you to implement logic that can process requests and responses globally across your web application. Middleware functions as a series of hooks that are executed during the request-response cycle, enabling you to perform actions like authentication, logging, modifying headers, and more. Here’s a step-by-step guide on how to create custom middleware in Django:
- Create a Python Module: Start by creating a Python module for your custom middleware in your Django app. You can name the module whatever you prefer, but it should have a clear and meaningful name. For example, you can create a file named `custom_middleware.py`.
- Define Middleware Classes: Inside the module, define one or more middleware classes. Each class should implement one or both of the following methods, depending on whether it handles request or response processing:
– `def __init__(self, get_response)`: This method is called when the middleware is initialized. It takes `get_response` as a parameter, which is a function used to get the response for a given request.
– `def __call__(self, request)`: This method is called for each incoming request. You can implement your logic here. Make sure to call `get_response(request)` to pass the request to the next middleware or view.
- Register Middleware: To use your custom middleware, add its fully-qualified Python import path to the `MIDDLEWARE` setting in your project’s `settings.py` file. The order in which middleware is listed matters, as it determines the order in which they are executed.
- Implement Middleware Logic: Within your middleware class, implement the logic you need. You can perform tasks such as modifying the request or response, logging information, adding headers, checking authentication, or even redirecting requests.
- Testing and Debugging: Thoroughly test your custom middleware to ensure it behaves as expected. You can use Django’s development server and the Django Debug Toolbar to help with debugging and profiling.
- Documentation: Always document your custom middleware, explaining its purpose, usage, and any configuration options it provides.
Creating custom middleware in Django is a powerful way to extend the framework’s functionality and implement cross-cutting concerns in a modular and reusable manner. Whether it’s adding security headers, handling authentication, or logging requests, custom middleware allows you to customize the behavior of your Django application to meet your specific requirements.
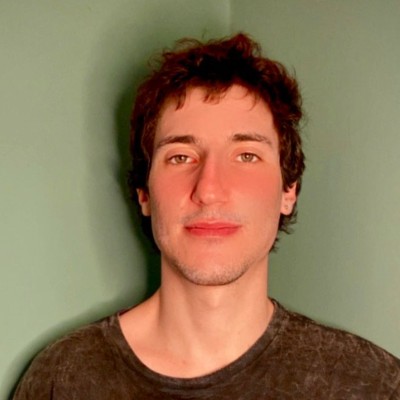
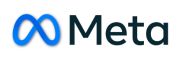