How to create models in Django?
Creating models in Django is a fundamental step in designing your application’s data structure. Models define the structure of your database tables, including the fields and their relationships. Django’s Object-Relational Mapping (ORM) simplifies this process by allowing you to define models as Python classes. Here’s a step-by-step guide on how to create models in Django:
- Create a Django App (if not already created): Before you can create models, ensure you have a Django app within your project where you can define them. You can create a new app using the `python manage.py startapp appname` command.
- Define Your Models: Inside your app’s directory, open the `models.py` file. This is where you define your models. Each model is represented as a Python class that inherits from `django.db.models.Model`. You define fields as class attributes, specifying the field type and any additional options. For example:
```python from django.db import models class Person(models.Model): first_name = models.CharField(max_length=30) last_name = models.CharField(max_length=30) email = models.EmailField() birthdate = models.DateField() ```
In this example, we define a `Person` model with fields for first name, last name, email, and birthdate.
- Migrations: After defining your models, create migrations using the `makemigrations` command:
``` python manage.py makemigrations appname ```
This command generates migration files that capture the changes to your database schema based on your model changes.
- Apply Migrations: Apply the migrations to create or update the database tables associated with your models:
``` python manage.py migrate ```
Django will automatically create the database tables according to your model definitions.
- Admin Integration (Optional): If you want to manage your model’s data through the Django admin panel, register your model in the app’s `admin.py` file. This allows you to easily add, edit, and delete model instances via the admin interface.
- Using Models in Views: In your views, you can interact with your models to retrieve, create, update, and delete data from the database using Django’s ORM.
Creating models in Django involves defining Python classes that represent your application’s data structure. These models define the database schema and allow you to interact with the database using Python code, abstracting away the underlying SQL complexity. Once you’ve created and applied migrations, your models are ready to store and manage data for your Django application.
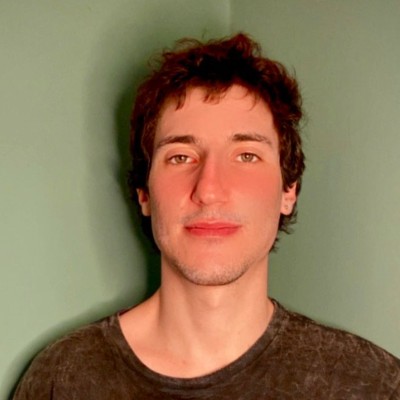
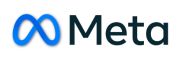