How to create a RESTful API with Django Rest Framework for a mobile app?
Creating a RESTful API with Django Rest Framework (DRF) for a mobile app is a common task, allowing your mobile app to communicate with a backend server seamlessly. Here’s a step-by-step guide on how to achieve this:
- Set Up Your Django Project:
Begin by creating a new Django project or using an existing one. Ensure you have DRF installed by adding it to your project’s `requirements.txt` or using `pip install djangorestframework`.
- Create a Django App:
Create a Django app within your project to organize your API-related code. You can do this using the command `python manage.py startapp yourappname`.
- Define Your Models:
Design your data models in Django using Django’s ORM. These models represent the data your API will interact with. Define models in your app’s `models.py` file.
- Create Serializers:
Serializers in DRF convert complex data types (such as Django model instances) into Python datatypes, making it easy to render them into JSON. Create serializers in your app’s `serializers.py` file to specify how your data should be serialized.
- Design Views:
Create views in your app’s `views.py` file to define the API’s behavior. You can use DRF’s class-based views or function-based views. Views handle incoming HTTP requests and return HTTP responses.
- Set Up URLs:
Configure URL patterns in your app’s `urls.py` file to map URLs to the views you created. Use DRF’s `router` for automatic URL routing whenever possible.
- Authentication and Permissions:
Decide on the authentication method you want to use (e.g., token-based authentication, JWT) and configure it in your project’s settings. Define permissions to control who can access various API endpoints.
- Testing:
Write tests for your API endpoints using DRF’s testing utilities. Testing ensures your API functions correctly and helps prevent regressions.
- Documentation:
Consider generating API documentation using tools like DRF’s built-in documentation or Swagger. Documenting your API makes it easier for mobile app developers to understand and use it.
- Middleware and Optimization:
Implement any necessary middleware for your API, such as CORS middleware for cross-origin requests. Optimize your API for performance and scalability.
- Deployment:
Deploy your Django project to a web server or cloud platform of your choice. Configure your server to serve the API over HTTPS for security.
- Monitoring and Maintenance:
Set up monitoring tools to keep an eye on your API’s performance and usage. Regularly maintain and update your API as needed.
- Versioning:
Consider implementing versioning for your API to ensure backward compatibility as you make changes and enhancements.
By following these steps, you can create a robust and RESTful API using Django Rest Framework, ready to serve data to your mobile app. This approach provides a structured and organized way to build APIs that can easily evolve and scale with your app’s needs.
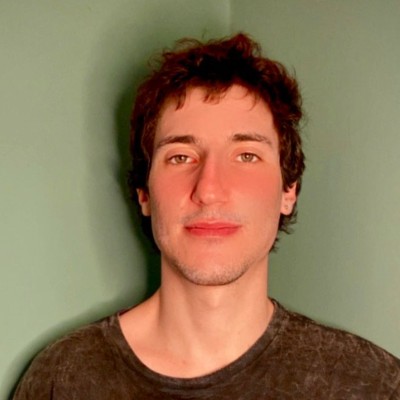
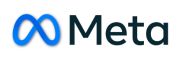