How to create URLs and views in Django?
Creating URLs and views in Django is a fundamental aspect of building web applications. URLs define the structure of your application’s URLs, while views handle the logic and data processing for each URL. Here’s a step-by-step guide on how to create URLs and views in Django:
Creating URLs:
- Define URL Patterns: To define URLs for your Django project, open the app’s `urls.py` file or create one if it doesn’t exist. In this file, you’ll define URL patterns using regular expressions or path converters. For example:
```python from django.urls import path from . import views urlpatterns = [ path('', views.index, name='index'), path('about/', views.about, name='about'), ] ```
In this example, we have defined two URL patterns: an empty string for the homepage and ‘/about/’ for an about page.
- Include App URLs in Project URLs: To make these URL patterns accessible to your project, include the app’s URL patterns in the project’s main `urls.py` file using the `include` function:
```python from django.contrib import admin from django.urls import include, path urlpatterns = [ path('admin/', admin.site.urls), path('', include('myapp.urls')), # Include the app's URL patterns ] ```
Creating Views:
- Define View Functions: Views are Python functions that handle the logic for rendering web pages. Create view functions in your app’s `views.py` file. Each view function takes a request as an argument and returns an HTTP response. For example:
```python from django.http import HttpResponse def index(request): return HttpResponse("Welcome to the homepage!") def about(request): return HttpResponse("This is the about page.") ```
- Mapping URLs to Views: In your app’s `urls.py` file, map each URL pattern to its corresponding view function using the `path()` function. The `name` parameter provides a unique identifier for the URL pattern, which is useful for generating URLs in templates and redirecting within your application.
- Template Rendering: Inside your view functions, you can render HTML templates by using the `render()` function, which combines a template and context data to generate an HTTP response. For example:
```python from django.shortcuts import render def index(request): return render(request, 'myapp/index.html', {'message': 'Welcome to the homepage!'}) ```
- Context Data: You can pass context data as a dictionary to your template, making it dynamic and allowing you to display data from your views in your HTML templates.
Creating URLs and views in Django involves defining URL patterns in your app’s `urls.py` file and associating them with view functions in the same file. View functions handle the logic and data processing, returning an HTTP response. By following this pattern, you can create a structured and organized web application with Django.
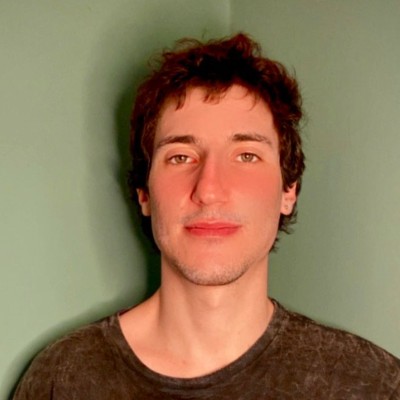
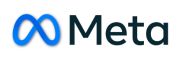