Mastering Multi-Platform Development with Django & Electron
Django, the high-level Python web framework, is known for its “batteries-included” philosophy, meaning that it provides a wide array of functionalities right out of the box. Initially, developers primarily used Django to create web applications. However, with the advent of new technologies and techniques, the horizon for Django has expanded, allowing for cross-platform app development. This means that the power of Django can now be harnessed to write applications that run on various platforms such as web, mobile, and desktop.
Table of Contents
This article dives into the realm of cross-platform development with Django and explores how developers can create apps that cater to multiple platforms simultaneously.
1. Django for Web Applications
The foundational strength of Django lies in web development. Let’s start by quickly brushing up on this.
Example: Creating a basic blog application.
```python # models.py from django.db import models class Blog(models.Model): title = models.CharField(max_length=200) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True) ``` ```python # views.py from django.shortcuts import render from .models import Blog def blog_list(request): blogs = Blog.objects.all() return render(request, 'blog_list.html', {'blogs': blogs}) ```
This is a simple illustration of a blog app where users can see a list of blog posts.
2. Django with React Native for Mobile Applications
React Native, developed by Facebook, allows developers to build native mobile apps using JavaScript and React. With the DRF (Django Rest Framework), Django can be combined with React Native to create dynamic mobile applications.
Example: Creating a mobile interface for the blog application.
Firstly, set up DRF to provide an API endpoint for your blogs:
```python # serializers.py from rest_framework import serializers from .models import Blog class BlogSerializer(serializers.ModelSerializer): class Meta: model = Blog fields = ['id', 'title', 'content', 'created_at'] ```
Now, create an API endpoint:
```python # views.py from rest_framework import viewsets from .models import Blog from .serializers import BlogSerializer class BlogViewSet(viewsets.ModelViewSet): queryset = Blog.objects.all().order_by('-created_at') serializer_class = BlogSerializer ```
In React Native, fetch the data from this endpoint:
```javascript fetch('https://yourdomain.com/api/blogs/') .then(response => response.json()) .then(data => this.setState({ blogs: data })); ```
3. Django with Electron for Desktop Applications
Electron allows for the creation of desktop applications using web technologies. To integrate Django with Electron, developers can package the Django app inside the Electron app.
Example: Creating a desktop app for the blog.
First, you’ll need to ensure that your Django app is running on a local server. Then, in your Electron main.js file:
```javascript const { app, BrowserWindow } = require('electron'); let mainWindow; function createWindow() { mainWindow = new BrowserWindow({width: 800, height: 600}); mainWindow.loadURL('http://localhost:8000/'); } app.on('ready', createWindow); ```
Here, Electron serves the Django app that’s running on the local server.
4. Challenges in Cross-Platform Development with Django
- Performance Concerns: While combining Django with platforms like React Native or Electron brings about cross-platform capabilities, it may also introduce performance overheads.
- Complexity: Managing multiple platforms means that developers need to be familiar with the intricacies of each platform.
- Syncing Issues: If you’re updating your backend (Django) or frontend (React Native/Electron), ensure that the changes are compatible across platforms.
5. Benefits of Cross-Platform Development with Django
- Code Reusability: Much of the backend code can be reused across different platforms, which saves development time.
- Consistent User Experience: By using a single backend, you can ensure consistent data and functionalities across platforms.
- Cost-Efficient: It’s often more cost-effective to develop for multiple platforms simultaneously than to develop separately for each one.
Conclusion
Django, initially a web-only framework, has proven its versatility by stepping into the realm of cross-platform development. By pairing Django with tools like React Native and Electron, developers can create applications that reach a wider audience spanning across web, mobile, and desktop platforms.
However, while the promise of cross-platform development is appealing, it’s crucial to approach it with a clear understanding of its challenges. Balancing the benefits against the complexities will ensure that you deliver a robust and high-performing application across all desired platforms.
Table of Contents
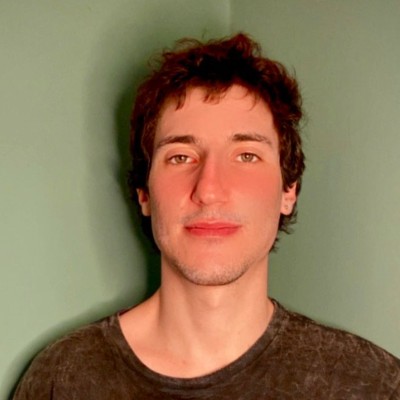
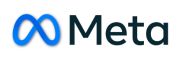