XSS Protection in Django: Essential Techniques for Secure Web Applications
In the realm of web development, security is paramount. As Django developers, one of our main concerns is protecting applications against Cross-Site Scripting (XSS) attacks. XSS is a prevalent security vulnerability that allows attackers to inject malicious scripts into webpages viewed by other users. This article will delve into the mechanisms Django provides to safeguard against XSS and demonstrate how to implement them effectively. You can hire Django Developers for your projects to ensure greater success.
Table of Contents
1. Understanding XSS in the Context of Django
Cross-Site Scripting attacks occur when an application includes untrusted data in a webpage without proper validation or escaping. This untrusted data can be executed as code in the browser, leading to a myriad of security issues, including session hijacking and personal data theft.
Django, being a high-level Python web framework, promotes clean, pragmatic design and emphasizes the importance of security in web applications. It provides several built-in features to prevent XSS attacks.
2. Auto-Escaping Template System
Django’s template system automatically escapes variables rendered in templates. This means that if your application uses Django’s template engine to display user-generated content, it is automatically protected against the most common XSS attacks.
For example:
```python from django.shortcuts import render def show_message(request): user_message = request.GET.get('message', 'Hello, World!') return render(request, 'message_display.html', {'message': user_message}) ```
In `message_display.html`:
```html <p>User Message: {{ message }}</p> ```
Here, any HTML in `user_message` will be escaped and rendered as text, not HTML.
3. Safe Template Filters
Despite the auto-escaping, there might be scenarios where you need to mark certain content as safe HTML. Django provides a `safe` filter for this purpose. However, use it cautiously as it opens up the potential for XSS if misused.
For example, if you trust a string of HTML and wish to render it as HTML, you can do:
```html {{ trusted_html_content|safe }} ```
4. Use of CSRF Tokens
Cross-Site Request Forgery (CSRF) is another security threat closely related to XSS. Django combats CSRF by using a token in POST forms. This token ensures that the form is submitted by an authenticated user, not by a third-party site.
Example:
```html <form method="post"> {% csrf_token %} ... </form> ```
5. Implementing Additional Security Measures
While Django offers robust protection against XSS, additional measures can be taken for enhanced security.
5.1. Content Security Policy (CSP)
Implementing a Content Security Policy can significantly increase your application’s resilience against XSS. CSP is a browser-side mechanism that allows you to specify the domains from which a browser should accept scripts. To implement CSP in Django, you can use middleware like `django-csp`.
5.2. Regular Security Audits
Regularly auditing your code for security vulnerabilities is crucial. Tools like OWASP ZAP and Django’s own `check` framework can help identify potential security issues.
6. Real-World Examples and Case Studies
For an in-depth look at how XSS vulnerabilities can impact applications, refer to case studies like The XSS vulnerability and how it was exploited.
Further, explore Django’s security documentation Django Project Security for a comprehensive understanding of Django’s security mechanisms.
Conclusion
In conclusion, while Django provides several layers of security against XSS attacks, it is the responsibility of developers to implement these features properly and remain vigilant. Keeping your Django applications secure is an ongoing process that involves staying updated with the latest security practices and continually auditing your applications for potential vulnerabilities.
Stay informed and proactive about web application security with resources like OWASP’s XSS Prevention Cheat Sheet and Django’s security documentation.
You can check out our other blog posts to learn more about Django. We bring you a complete guide titled Django Real-time Alerts: A Push Notification Guide along with the Master Real-Time Communication in Django Apps with WebSockets and The Essential Guide to Social Logins in Django with OAuth and OpenID which will help you understand and gain more insight into the Django programming language.
Table of Contents
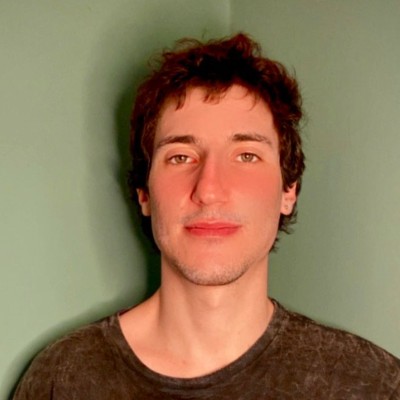
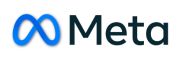