How can I create custom template tags and filters in Django?
Creating custom template tags and filters in Django allows you to extend the functionality of the template language to meet your specific application needs. Custom template tags are used for more complex template logic, while custom filters are used to modify the output of template variables. Here’s how you can create custom template tags and filters in Django:
Creating Custom Template Tags:
- Folder Structure: Start by creating a Python package named “templatetags” within one of your app directories. This package will hold your custom template tags.
- Python Module: Inside the “templatetags” package, create a Python module (e.g., `custom_tags.py`) where you’ll define your custom template tags.
- Import Requirements: In the Python module, import necessary modules and classes. For custom template tags, you typically need to import `template` from `django` and create an instance of `template.Library()`.
- Define Tag Functions: Write your custom template tag functions within the Python module. These functions should take at least one argument (usually the template context) and return the result of your custom logic.
- Register the Tags: Use the `register` object to register your custom template tag functions. For example:
```python from django import template register = template.Library() @register.simple_tag def my_custom_tag(context, arg1, arg2): # Your tag logic here return result ```
Creating Custom Filters:
- Folder Structure: Similar to custom tags, create a “templatetags” package within your app’s directory.
- Python Module: Inside the “templatetags” package, create a Python module (e.g., `custom_filters.py`) for your custom filters.
- Import Requirements: In the Python module, import necessary modules and classes. For custom filters, you typically need to import `template` from `django` and create an instance of `template.Library()`.
- Define Filter Functions: Write your custom filter functions within the Python module. These functions should take at least one argument (the input value) and return the modified output.
- Register the Filters: Use the `register` object to register your custom filter functions. For example:
```python from django import template register = template.Library() @register.filter def my_custom_filter(value, arg): # Your filter logic here return modified_value ```
Using Custom Tags and Filters:
After creating your custom template tags and filters, you can use them in your templates by loading them at the top of the template file using the `{% load %}` template tag. For example:
```html {% load custom_tags custom_filters %} ```
Then, you can use your custom tags and filters just like built-in ones within your template code:
```html {% my_custom_tag arg1 arg2 %} {{ some_variable|my_custom_filter:arg }} ```
By following these steps, you can create and use custom template tags and filters in Django to add specific template logic and data transformations tailored to your application’s requirements.
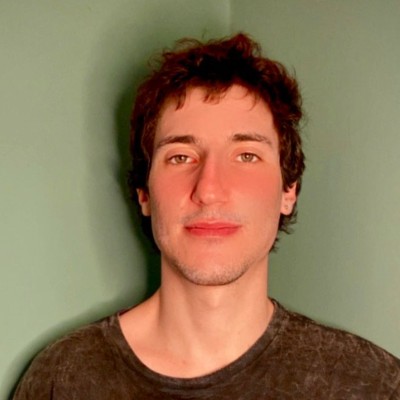
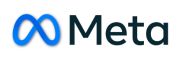