Django and Data Encryption: Securing Sensitive Information in Your App
In today’s digital age, the importance of securing sensitive data cannot be overstated. Whether you’re building a social media platform, an e-commerce site, or a healthcare application, protecting user information is paramount. Django, a high-level Python web framework, offers powerful tools for developing secure web applications, including built-in features for data encryption. In this blog post, we’ll delve into the world of data encryption in Django, exploring why it’s crucial and how to implement it effectively.
Table of Contents
1. Why Data Encryption Matters
1.1. The Stakes are High
Sensitive data such as user passwords, personal information, and payment details are prime targets for cybercriminals. A security breach can lead to severe consequences, including legal issues, loss of trust, and financial damage. Data breaches have become increasingly common, emphasizing the urgency of implementing robust security measures.
1.2. Legal and Ethical Obligations
Various data protection regulations, such as the General Data Protection Regulation (GDPR) and the Health Insurance Portability and Accountability Act (HIPAA), impose legal obligations on businesses to safeguard user data. Non-compliance can result in substantial fines and reputational damage.
1.3. User Trust
Users entrust businesses with their information, expecting it to remain confidential. Building and maintaining this trust is essential for the success of your application. Implementing data encryption demonstrates your commitment to protecting user privacy.
2. Django’s Built-In Encryption Tools
Django simplifies the process of data encryption by providing built-in tools and libraries. Let’s explore some of these features and best practices for securing sensitive information in your Django application.
2.1. Using Django’s Secret Key
Django uses a secret key to handle various security-related tasks, including hashing and signing cookies and data. This secret key should remain confidential and should never be shared or exposed in your codebase. You can define it in your project’s settings like this:
python # settings.py SECRET_KEY = 'your_secret_key_here'
Make sure to generate a strong, unique key for each Django project to enhance security.
2.2. Protecting Passwords with Argon2
Django employs the Argon2 password hashing algorithm by default, which is a robust choice for securing user passwords. When a user registers or logs in, Django automatically hashes and verifies passwords, ensuring that plain-text passwords are never stored in the database. Here’s an example of how to create a password hash:
python from django.contrib.auth.hashers import make_password password = 'user_password' hashed_password = make_password(password)
2.3. Encrypting Database Fields
Django also allows you to encrypt specific fields in your database models using the EncryptedCharField and EncryptedTextField from the django-encrypted-fields package. This ensures that even if your database is compromised, the sensitive data within these fields remains secure.
python from encrypted_fields import EncryptedCharField from django.db import models class UserProfile(models.Model): username = models.CharField(max_length=50) credit_card = EncryptedCharField(max_length=16)
2.4. Using HTTPS for Secure Communication
Encrypting data at rest is crucial, but data in transit is equally vulnerable. Implementing HTTPS ensures that data transmitted between the client and server is encrypted. You can configure HTTPS easily using Django and a trusted SSL certificate. Here’s a basic setup using Django’s built-in development server:
python # settings.py SECURE_PROXY_SSL_HEADER = ('HTTP_X_FORWARDED_PROTO', 'https') SESSION_COOKIE_SECURE = True CSRF_COOKIE_SECURE = True
For production, consider using a web server like Nginx or Apache to handle SSL termination.
3. Best Practices for Data Encryption in Django
While Django provides excellent tools for data encryption, following best practices is crucial to maximize security. Here are some additional tips:
3.1. Regularly Update Dependencies
Keep your Django version and third-party packages up-to-date to ensure you’re protected against known vulnerabilities. Django’s security team actively monitors and patches security issues.
bash pip install --upgrade django
3.2. Implement Two-Factor Authentication (2FA)
Encourage users to enable two-factor authentication for their accounts. Django offers packages like django-otp and django-otp-plugins to simplify 2FA implementation.
3.3. Monitor and Audit
Set up monitoring and logging to detect suspicious activities. Tools like Django Debug Toolbar can help you track performance and potential security issues during development.
python # settings.py MIDDLEWARE += ['debug_toolbar.middleware.DebugToolbarMiddleware'] INTERNAL_IPS = ['127.0.0.1']
3.4. Use Third-Party Security Libraries
Explore third-party libraries that enhance Django’s security features. Popular options include django-allauth for authentication, django-rest-framework for API security, and django-cors-headers for handling Cross-Origin Resource Sharing (CORS).
4. Data Encryption Beyond Django
While Django provides robust security features, a comprehensive security strategy involves more than just server-side measures. Consider the following practices to bolster your application’s overall security:
4.1. Client-Side Encryption
Implement client-side encryption to ensure data remains secure even before it reaches your server. JavaScript libraries like CryptoJS and Web Crypto API can help you achieve this.
4.2. Regular Security Audits
Perform regular security audits and penetration testing to identify and address vulnerabilities proactively.
4.3. Employee Training
Educate your development team about security best practices to minimize the risk of accidental data exposure.
4.4. Secure APIs
If your application includes APIs, secure them using authentication tokens, rate limiting, and input validation.
Conclusion
Data encryption is a fundamental component of any robust security strategy for your Django application. By utilizing Django’s built-in encryption tools, following best practices, and extending your security efforts beyond Django, you can create a safer environment for sensitive user data. Remember, safeguarding your users’ information is not just a legal requirement; it’s a trust-building exercise that can set your application apart in an increasingly competitive digital landscape.
Table of Contents
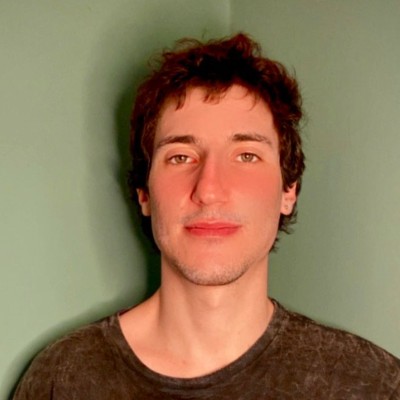
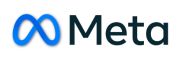