Elevate Your Django Apps with Real-Time Data Analysis Capabilities
The world of web development and data science often seem like two separate entities. However, with the rise in demand for real-time data analysis and visualization, there’s an increasing need to integrate data science capabilities into web applications. Django, a high-level Python web framework, is an excellent tool to bridge this gap.
Table of Contents
In this article, we’ll dive into how Django can be utilized in the realm of data science, focusing on data analysis and visualization. We’ll walk through a few examples, and by the end, you’ll have a clear understanding of how Django fits into the data science ecosystem.
1. Setting up Django with Pandas and Matplotlib
Before diving into examples, ensure that you have Django set up. Additionally, for data analysis and visualization, we’ll be using Pandas and Matplotlib. Install them via pip:
```bash pip install django pandas matplotlib ```
2. Incorporating Pandas with Django Models
Let’s consider a Django model for storing sales data:
```python from django.db import models class Sale(models.Model): product_name = models.CharField(max_length=255) units_sold = models.PositiveIntegerField() sale_date = models.DateField() ```
With a populated database, you can easily convert Django QuerySets into Pandas DataFrames:
```python import pandas as pd from .models import Sale def sales_to_dataframe(): queryset = Sale.objects.all().values() df = pd.DataFrame.from_records(queryset) return df ```
3. Analyzing Data
With your data in a DataFrame, you can quickly perform data analysis operations. For instance, to get the total sales per product:
```python def total_sales_per_product(df): return df.groupby('product_name').units_sold.sum() ```
4. Visualizing Data with Matplotlib
Once the data is analyzed, you might want to visualize it. Let’s visualize the total sales per product:
```python import matplotlib.pyplot as plt def plot_sales_per_product(df): sales = total_sales_per_product(df) sales.plot(kind='bar', title='Total Sales per Product') plt.ylabel('Units Sold') plt.show()
5. Embedding Visualizations in Django Views
To embed a Matplotlib visualization in a Django view, you can save the plot as an image and serve it through Django:
```python import io from django.http import FileResponse def sales_plot_view(request): df = sales_to_dataframe() buf = io.BytesIO() plot_sales_per_product(df) plt.savefig(buf, format='png') buf.seek(0) return FileResponse(buf, as_attachment=True, filename='sales_plot.png') ```
Add the appropriate URL and template to display the plot within your Django application.
6. Real-time Data Analysis with Django Channels
For real-time analysis, Django Channels comes in handy. With Channels, you can use WebSockets to push updated analysis and visualizations to your users without requiring a page refresh.
For example, if you want to push the sales analysis every time a new sale is recorded:
- Set up Django Channels and integrate it with your project.
- Modify the Sale model’s save method to trigger the real-time update.
- Use a WebSocket consumer to push updates to the connected clients.
7. Integrating Advanced Analysis Tools
Django’s Python nature allows seamless integration with other data analysis tools like scikit-learn, TensorFlow, or Statsmodels. For instance, you could train a predictive model on your sales data to forecast future sales and integrate the prediction results directly into your Django app.
Conclusion
Django, with its robust and scalable architecture, offers a powerful platform to integrate data science capabilities. The synergy between Django and tools like Pandas and Matplotlib makes it straightforward to analyze and visualize data in a web context.
By incorporating data science into Django apps, you can provide your users with insightful data visualizations, real-time analysis, and predictive capabilities. As the world becomes more data-driven, Django stands out as a prime candidate for building integrated, data-rich web applications.
Table of Contents
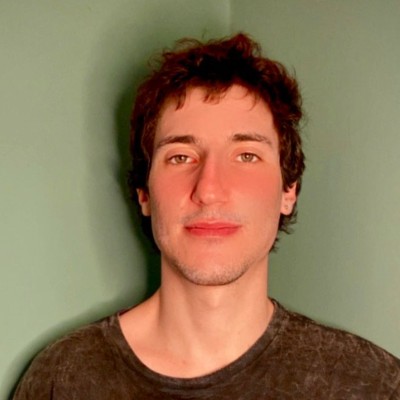
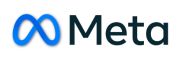