How to implement data validation and serialization in Django REST framework?
In Django REST framework (DRF), data validation and serialization play a crucial role in ensuring that data is received, processed, and sent in a consistent and safe manner. Here’s a comprehensive guide on how to implement data validation and serialization in DRF:
- Serializer Classes:
Serializer classes in DRF define the structure of the incoming and outgoing data. They convert complex data types, such as Django querysets and model instances, into native Python data types that can be easily rendered into JSON, XML, or other content types.
- ModelSerializer:
When working with Django models, you can use the `ModelSerializer` class, which automatically generates serializer fields based on the model fields. This simplifies the process of creating serializers for your models.
- Validation:
DRF provides built-in validation for serializer fields. You can define validation rules for fields in the serializer class using methods like `validate_<field_name>` or by adding validators to individual fields.
- Custom Validation:
If you need more complex validation logic, you can override the `validate` method in your serializer class. This method can perform validation that involves multiple fields and raise `serializers.ValidationError` when validation fails.
- Deserialization:
When data is received from a client, DRF automatically deserializes it based on the serializer class. During deserialization, input data is converted into Python data types and validated. If validation fails, the serializer raises an exception.
- Serialization:
When you return data to the client, DRF serializes it based on the serializer class. This ensures that data is presented in the format expected by the client, whether it’s JSON, XML, or another format.
- Response Validation:
It’s essential to validate data before sending it to the client to ensure it meets any client-specific requirements. Use serializer fields like `serializers.DecimalField` or `serializers.ChoiceField` to define how data should be serialized.
- Custom Serializers:
In cases where you need custom serialization logic, you can create custom serializer classes by inheriting from `serializers.Serializer`. This allows you to define the exact structure of your serialized data.
- Testing:
Thoroughly test your serializers to ensure they handle validation and serialization correctly. DRF provides testing utilities for this purpose.
By following these steps, you can effectively implement data validation and serialization in Django REST framework. This ensures that data exchanged between your API and clients is accurate, safe, and compliant with your application’s business rules.
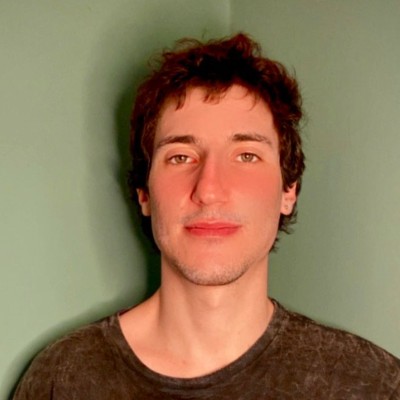
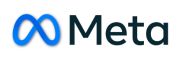