Uncover Patterns and Trends with Django’s Data Visualization Tools
Data visualization is an essential part of data analysis. It facilitates a clear understanding of the data by portraying it in a visual context to uncover patterns, trends, and insights. This skill set is also a key reason why many businesses choose to hire Django developers. This article will focus on how to integrate data visualization within a Django application, a task that professional Django developers excel at, using popular libraries like Matplotlib, Pandas, and Bokeh.
Table of Contents
1. Django: An Overview
Django is a high-level, Python-based framework that enables rapid development of secure and maintainable websites. It is built by skilled developers and incorporates a vast number of features, enabling developers to concentrate on the unique aspects of their application, rather than reimplementing common functionalities.
2. Data Visualization: A Brief
Data visualization is the presentation of data in a pictorial or graphical format. It enables decision-makers to visualize data analytics presented visually, so they can grasp difficult concepts or identify new patterns. With interactive visualization, you can take the concept a step further by using technology to drill down into charts and graphs for more detail.
3. Setting Up Django
To begin, we’ll need a Django environment. Assuming you have Python installed, you can install Django via pip:
```bash pip install django ```
Create a new Django project named ‘myproject’:
```bash django-admin startproject myproject ```
Move to your project directory and start a new app called ‘myapp’:
```bash cd myproject django-admin startapp myapp ```
Register your new application within the `INSTALLED_APPS` in your `settings.py` file.
4. Visualizing Data with Matplotlib and Pandas
4.1 Install Dependencies
First, we need to install Matplotlib and Pandas:
```bash pip install matplotlib pandas ```
4.2 Fetching and Cleaning Data
Assume we have a CSV file named ‘sales.csv’ containing data for the sales records. We can use pandas to read this data and clean it if necessary.
```python import pandas as pd data = pd.read_csv('sales.csv') ```
4.3 Plotting the Data
Let’s plot a simple bar chart showing sales over time:
```python import matplotlib.pyplot as plt data.plot(kind='bar', x='date', y='sales') plt.show() ```
The `plot()` function is a wrapper function in pandas, which is called `matplotlib.pyplot.plot()`. The parameters ‘x’ and ‘y’ take column names, and ‘kind’ refers to the type of graph.
4.4 Integrating with Django
Integrating the graph into a Django application requires generating a plot, saving it as an image, and then serving the image through Django.
In your `views.py`:
```python import matplotlib.pyplot as plt import pandas as pd from django.shortcuts import render from django.conf import settings from django.http import HttpResponse import os def plot(request): data = pd.read_csv('sales.csv') data.plot(kind='bar', x='date', y='sales') fig = plt.gcf() fig.savefig('myapp/static/myapp/sales.png') return render(request, 'myapp/plot.html') ```
In your `myapp/plot.html`:
```html <img src="{% static 'myapp/sales.png' %}"> ```
The generated graph will be available at your application’s `/plot` route.
5. Creating Interactive Graphs with Bokeh
5.1 Install Bokeh
Install Bokeh using pip:
```bash pip install bokeh ```
5.2 Creating an Interactive Plot
With Bokeh, we can create interactive plots that can be embedded in Django templates. Below, we generate a line plot of sales over time.
```python from bokeh.plotting import figure from bokeh.embed import components def plot(request): data = pd.read_csv('sales.csv') p = figure(title="Sales Over Time", x_axis_label='Date', y_axis_label='Sales') p.line(data['date'], data['sales'], legend_label="Sales", line_width=2) script, div = components(p) return render(request, 'myapp/plot.html', {'script': script, 'div': div}) ```
In your `myapp/plot.html`:
```html {{ script|safe }} {{ div|safe }} ```
The `components()` function of Bokeh generates JavaScript and HTML code necessary to render the plot. We pass this to our Django template, where it gets embedded into the HTML.
Conclusion
Integrating data visualization into Django applications is an excellent way to display your data in an easy-to-understand and interactive manner. By using libraries like Matplotlib, Pandas, and Bokeh, you can create a wide variety of static and interactive plots to suit your needs. If the process seems overwhelming, hiring Django developers could be an efficient solution.
It’s crucial to remember that while visuals are engaging and informative, they should also be used responsibly. Always ensure that your visualizations accurately represent your data and do not mislead the audience.
Data visualization can significantly improve your application by providing insight into patterns, trends, and correlations that might go unnoticed in text-based data. This, in turn, can lead to better decision-making and provide a competitive edge to your business or research. Whether you decide to tackle this project on your own or hire Django developers, the investment in data visualization is sure to pay off. Happy coding!
Table of Contents
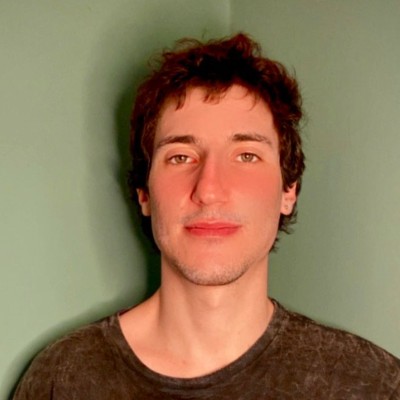
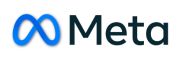